如何使用Python编写一个包括图片爬取在内的功能全面的网络爬虫程序?
时间: 2024-10-20 16:16:22 浏览: 30
在Python中,你可以使用一系列库来构建一个包含图片爬取功能的网络爬虫程序,比如`requests`, `beautifulsoup4`, `pandas`, 和 `selenium`等。这里我会给你一个基础的步骤:
1. **安装必要的库**:
首先确保已经安装了以下库(如果还没有的话):
```
pip install requests beautifulsoup4 pandas pillow selenium (如果需要处理动态网页)
```
2. **获取HTML内容**:
使用`requests`库发送HTTP请求并获取网页源代码。例如,抓取一个URL:
```python
import requests
url = "https://www.example.com"
response = requests.get(url)
html_content = response.text
```
3. **解析HTML**:
使用`BeautifulSoup`解析HTML内容,提取图片链接。如果你的网页结构复杂,可能需要用到CSS选择器或XPath表达式:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
img_tags = soup.find_all('img') # 这里假设所有的图片都是用<img>标签表示
img_links = [img['src'] for img in img_tags]
```
4. **下载图片**:
使用`requests`下载图片到本地文件。这里假设图片都在同一域名下:
```python
import os
if not os.path.exists("images"):
os.makedirs("images")
for link in img_links:
image_response = requests.get(link, stream=True)
with open(f"images/{os.path.basename(link)}", 'wb') as out_file:
out_file.write(image_response.content)
```
5. **处理动态加载的内容**:
如果页面有JavaScript加载的图片,可以使用`selenium`模拟浏览器行为,如:
```python
from selenium import webdriver
driver = webdriver.Chrome() # 假设已安装ChromeDriver
driver.get(url)
# 等待图片完全加载
time.sleep(5) # 或者使用WebDriverWait
img_elements = driver.find_elements_by_tag_name('img')
img_links = [el.get_attribute('src') for el in img_elements]
# 同样下载图片...
```
6. **数据组织**:
可能的话,你可以使用`pandas`库来整理图片链接、下载状态或其他相关信息。
7. **错误处理和进度监控**:
添加适当的错误检查和日志记录,以及处理可能的网络连接问题。
记得遵守网站的robots.txt规则,尊重版权,并在适当的地方设置延迟,以免对目标服务器造成过大的压力。
阅读全文
相关推荐
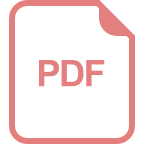
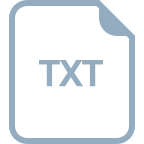
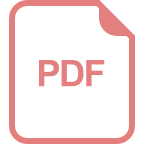
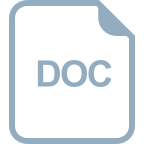

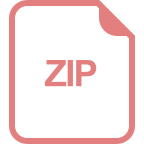
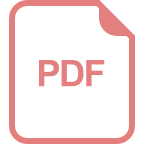
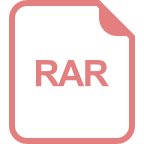
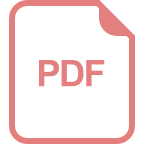
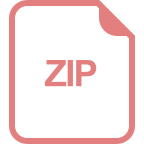
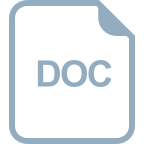
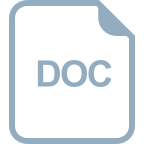
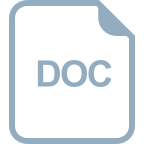
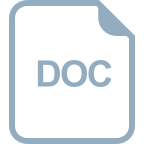
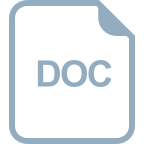
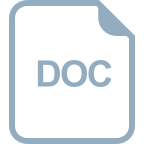
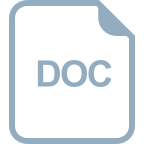

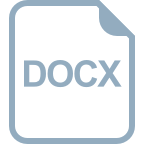