unity 写一个敌人攻击我方的脚本,要求每两秒瞄准我方(LookAt),发射子弹,子弹碰到我方时,扣掉我方的血量,生成爆炸特效,摧毁子弹,当我方死亡时,不能移动,不能开火
时间: 2024-12-11 21:26:52 浏览: 6
在Unity中编写一个这样的敌人攻击脚本,首先需要一些基本组件,如Rigidbody2D(用于物理碰撞)、AudioSource(声音效果)、Animator(动画控制)等。以下是一个简单的C#脚本示例:
```csharp
using UnityEngine;
using System.Collections;
public class EnemyAttack : MonoBehaviour
{
public Transform target; // 我方角色的Transform
public GameObject bulletPrefab; // 子弹的游戏对象
public float shootInterval = 2f; // 发射间隔时间
private Rigidbody2D rb;
private Animator anim;
private bool isFiring = false;
void Start()
{
rb = GetComponent<Rigidbody2D>();
anim = GetComponent<Animator>();
}
void Update()
{
LookAtTarget();
if (!isFiring && Time.timeSinceLevelLoad > shootInterval)
{
ShootBullet();
isFiring = true;
}
}
void FixedUpdate()
{
// 检测碰撞并处理血量减少和子弹销毁
Collider2D[] colliders = Physics2D.OverlapCircle(transform.position, bulletPrefab.GetComponent<Bullet>().radius);
foreach (Collider2D collider in colliders)
{
if (collider.CompareTag("Player"))
{
PlayerHealth playerHealth = collider.gameObject.GetComponent<PlayerHealth>();
if (playerHealth != null)
{
playerHealth.TakeDamage();
Destroy(collider.gameObject); // 消毁子弹
CreateExplosion(collider.position); // 生成爆炸特效
}
}
}
// 当我方生命值归零,停止敌人的动作
if (IsPlayerDead())
{
isFiring = false;
anim.SetTrigger("StopMovingAndFiring");
}
}
void LookAtTarget()
{
transform.LookAt(target);
}
void ShootBullet()
{
GameObject bulletInstance = Instantiate(bulletPrefab, transform.position, Quaternion.identity);
Rigidbody2D bulletRB = bulletInstance.GetComponent<Rigidbody2D>();
bulletRB.velocity = transform.forward * bulletsSpeed; // 设置子弹速度
}
bool IsPlayerDead()
{
return GetComponent<PlayerHealth>().Health <= 0;
}
void CreateExplosion(Vector3 position)
{
// 创建爆炸效果的代码...
}
}
```
这个脚本假设你已经有了一个名为`PlayerHealth`的组件来管理玩家的生命值,以及`Bullet`组件来表示子弹。
阅读全文
相关推荐
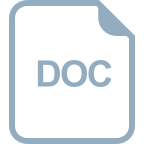
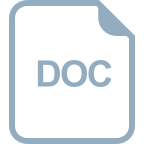
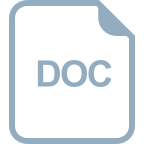
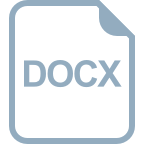
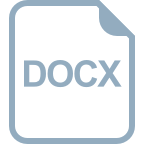
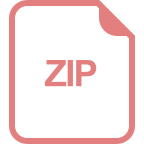
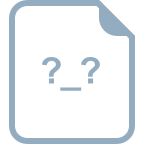
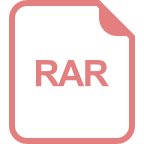
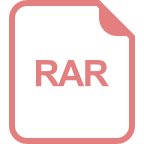
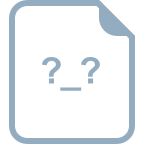
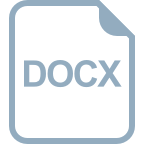