如何用stm32f103c8t6控制共阳极三位数码管
时间: 2024-05-18 10:10:01 浏览: 30
可以使用STM32F103C8T6的GPIO口来控制共阳极三位数码管显示数字。由于共阳极三位数码管需要同时驱动三个数码管,因此需要使用定时器中断来实现动态扫描显示。下面是一个简单的代码实现:
1.首先需要在STM32F103C8T6上配置GPIO口为输出,配置定时器中断扫描三个数码管。
```c
#include "stm32f10x.h"
#define uchar unsigned char
#define uint unsigned int
// 定义数码管端口,共阳极三位数码管需要同时输出三个数码管的段选和位选信号
#define DIG0_ON GPIOA->BRR = GPIO_Pin_0
#define DIG1_ON GPIOA->BRR = GPIO_Pin_1
#define DIG2_ON GPIOA->BRR = GPIO_Pin_2
#define DIG0_OFF GPIOA->BSRR = GPIO_Pin_0
#define DIG1_OFF GPIOA->BSRR = GPIO_Pin_1
#define DIG2_OFF GPIOA->BSRR = GPIO_Pin_2
#define SEG_A_ON GPIOB->BRR = GPIO_Pin_1
#define SEG_B_ON GPIOC->BRR = GPIO_Pin_5
#define SEG_C_ON GPIOC->BRR = GPIO_Pin_4
#define SEG_D_ON GPIOC->BRR = GPIO_Pin_3
#define SEG_E_ON GPIOC->BRR = GPIO_Pin_2
#define SEG_F_ON GPIOC->BRR = GPIO_Pin_1
#define SEG_G_ON GPIOC->BRR = GPIO_Pin_0
#define SEG_DP_ON GPIOD->BRR = GPIO_Pin_2
#define SEG_A_OFF GPIOB->BSRR = GPIO_Pin_1
#define SEG_B_OFF GPIOC->BSRR = GPIO_Pin_5
#define SEG_C_OFF GPIOC->BSRR = GPIO_Pin_4
#define SEG_D_OFF GPIOC->BSRR = GPIO_Pin_3
#define SEG_E_OFF GPIOC->BSRR = GPIO_Pin_2
#define SEG_F_OFF GPIOC->BSRR = GPIO_Pin_1
#define SEG_G_OFF GPIOC->BSRR = GPIO_Pin_0
#define SEG_DP_OFF GPIOD->BSRR = GPIO_Pin_2
// 数码管显示数据表,共阳极三位数码管需要同时输出三个数码管的段选和位选信号
uchar dis_table[16]={{0,0,0}, {1,0,0}, {0,1,0}, {1,1,0}, {0,0,1}, {1,0,1}, {0,1,1}, {1,1,1}, {0,0,0}, {1,0,0}, {0,1,0}, {1,1,0}, {0,0,1}, {1,0,1}, {0,1,1}, {1,1,1}};
// 定义定时器中断函数
void TIM1_UP_IRQHandler(void)
{
static uint cnt = 0;
static uint num_cnt = 0;
static uint num = {0,0,0};
if(TIM_GetITStatus(TIM1,TIM_IT_Update)!=RESET)
{
TIM_ClearITPendingBit(TIM1,TIM_IT_Update);
if(cnt == 0)
{
// 计算当前位数字和位选信号
num_cnt++;
if(num_cnt > 2) num_cnt = 0;
num[num_cnt] = num[num_cnt] + 1;
if(num[num_cnt] > 9) num[num_cnt] = 0;
// 输出数字和位选信号
DIG0_OFF;
DIG1_OFF;
DIG2_OFF;
SEG_A_OFF;
SEG_B_OFF;
SEG_C_OFF;
SEG_D_OFF;
SEG_E_OFF;
SEG_F_OFF;
SEG_G_OFF;
SEG_DP_OFF;
if(num_cnt == 0)
{
if(num != 0) SEG_A_ON;
SEG_B_ON;
if(num != 1 && num != 4) SEG_C_ON;
if(num != 0 && num != 1 && num != 7) SEG_D_ON;
if(num == 0 || num == 2 || num == 6 || num == 8) SEG_E_ON;
if(num != 1 && num != 2 && num != 3 && num != 7) SEG_F_ON;
if(num != 0 && num != 1 && num != 7) SEG_G_ON;
if(num == 0) SEG_DP_ON;
DIG0_ON;
}
else if(num_cnt == 1)
{
if(num != 0) SEG_A_ON;
if(num != 2 && num != 6 && num != 8 && num != 0) SEG_B_ON;
if(num != 1 && num != 4 && num != 7) SEG_C_ON;
if(num != 1 && num != 4) SEG_D_ON;
if(num != 0 && num != 1 && num != 7) SEG_E_ON;
if(num != 1 && num != 2 && num != 3 && num != 7 && num != 0) SEG_F_ON;
if(num != 0 && num != 1 && num != 7) SEG_G_ON;
DIG1_ON;
}
else if(num_cnt == 2)
{
if(num != 0) SEG_A_ON;
if(num != 2 && num != 6 && num != 8 && num != 0) SEG_B_ON;
if(num != 1 && num != 4 && num != 7) SEG_C_ON;
if(num != 1 && num != 4) SEG_D_ON;
if(num != 0 && num != 1 && num != 7) SEG_E_ON;
if(num != 1 && num != 2 && num != 3 && num != 7 && num != 0) SEG_F_ON;
if(num != 0 && num != 1 && num != 7) SEG_G_ON;
DIG2_ON;
}
}
cnt++;
if(cnt > 100) cnt = 0;
}
}
int main(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB | RCC_APB2Periph_GPIOC | RCC_APB2Periph_GPIOD, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3 | GPIO_Pin_4 | GPIO_Pin_5;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3 | GPIO_Pin_4 | GPIO_Pin_5;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOC, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOD, &GPIO_InitStructure);
// 定时器TIM2配置,设置周期为1ms
TIM_TimeBaseStructure.TIM_Period = 999;
TIM_TimeBaseStructure.TIM_Prescaler = 71;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseStructure.TIM_ClockDivision = 0;
TIM_TimeBaseStructure.TIM_RepetitionCounter = 0;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
// 定时器TIM2中断配置
NVIC_InitStructure.NVIC_IRQChannel = TIM2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
TIM_ITConfig(TIM2,TIM_IT_Update,ENABLE);
TIM_Cmd(TIM2,ENABLE);
while(1);
}
```
2.配置完成后,就可以通过修改num数组的值来改变三位数码管显示的数字了。
相关推荐
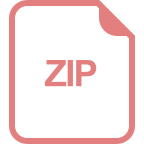
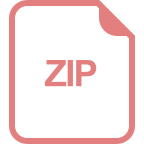
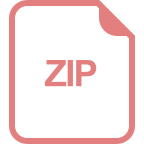










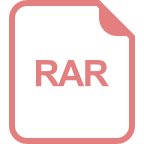
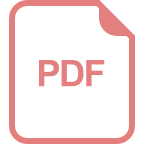
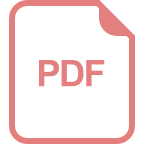