springboot发送post请求带json参数如何写
时间: 2024-12-23 09:23:28 浏览: 3
在Spring Boot中发送POST请求并携带JSON参数,通常需要使用`RestTemplate`、`Feign`、`Retrofit`或者`HttpClient`等库。以下是使用`RestTemplate`的一个简单示例:
```java
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.web.client.RestTemplate;
public class ExampleService {
private RestTemplate restTemplate = new RestTemplate();
public void sendPostRequest(String url, Object requestBody) {
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
// 将对象转换为JSON字符串
String json = new ObjectMapper()..writeValueAsString(requestBody);
// 发送POST请求
ResponseEntity<String> response = restTemplate.postForEntity(url, json, String.class);
System.out.println("Response Status: " + response.getStatusCode());
System.out.println("Response Body: " + response.getBody());
}
}
```
在这个例子中,`requestBody`是你想要发送的JSON对象,`ObjectMapper`来自Jackson库,用于序列化Java对象为JSON。
如果你想使用HTTP客户端直接操作,可以使用`httpclient4.x`库:
```java
import org.apache.http.entity.ContentType;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("http://example.com/api");
httpPost.setHeader(HttpHeaders.CONTENT_TYPE, ContentType.APPLICATION_JSON_VALUE);
StringEntity requestJson = new StringEntity(json, ContentType.APPLICATION_JSON);
httpPost.setEntity(requestJson);
CloseableHttpResponse response = httpClient.execute(httpPost);
try {
System.out.println("Response Status: " + response.getStatusLine());
System.out.println("Response Body: " + EntityUtils.toString(response.getEntity()));
} finally {
response.close();
httpClient.close();
}
```
阅读全文
相关推荐
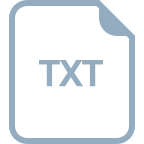
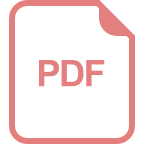
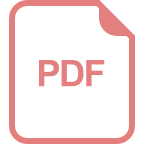




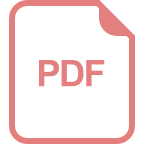
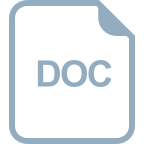









