HttpURLConnection 下载图片
时间: 2024-08-16 10:00:29 浏览: 38
在Java中,使用`HttpURLConnection`下载图片通常涉及到以下几个步骤:
1. 创建`URL`对象:首先,你需要创建一个`URL`对象,代表你想下载的图片地址。
```java
URL imageUrl = new URL("http://example.com/image.jpg");
```
2. 打开连接:使用`openConnection()`方法建立到服务器的连接,并将其转换为`HttpURLConnection`。
```java
HttpURLConnection conn = (HttpURLConnection) imageUrl.openConnection();
```
3. 设置请求方法和允许保存:这里通常我们设置请求方法为`GET`,并允许输入流(`InputStream`)以保存图片。
```java
conn.setRequestMethod("GET");
conn.setDoOutput(true); // 如果是POST请求则需设为false
conn.connect();
```
4. 获取输入流:检查连接状态,如果成功,则获取`InputStream`。
```java
int responseCode = conn.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
InputStream inputStream = conn.getInputStream();
} else {
throw new RuntimeException("Failed : HTTP error code : " + responseCode);
}
```
5. 写入文件:将输入流读取的内容写入本地文件。这一步可以使用`FileOutputStream`或者`BufferedOutputStream`。
```java
File file = new File("image.jpg");
FileOutputStream outputStream = new FileOutputStream(file);
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
outputStream.flush();
outputStream.close();
inputStream.close();
```
6. 关闭连接:最后别忘了关闭所有连接。
```java
conn.disconnect();
```
阅读全文
相关推荐
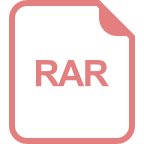
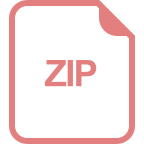
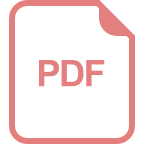
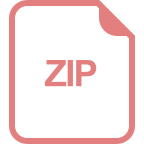
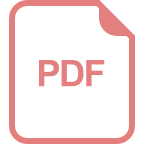
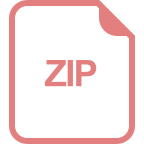
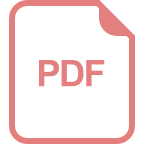
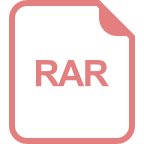
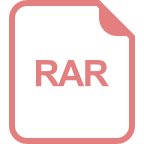
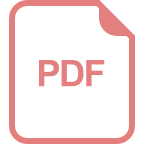
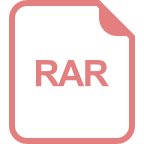
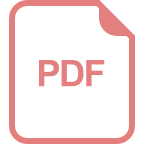
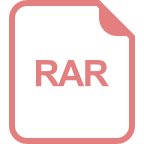
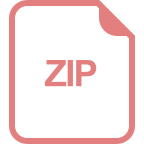
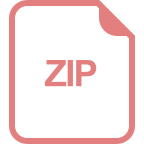
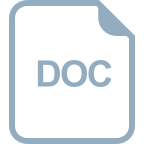