python range arange
时间: 2024-08-12 10:04:31 浏览: 33
在Python中,`range()` 和 `numpy.arange()` 都是用来生成一系列数字序列的函数,但它们之间有一些区别。
**range() 函数**:
这是内置的 Python 序列类型,用于创建一个整数序列,通常用于循环控制。`range(stop)`、`range(start, stop)` 或者 `range(start, stop, step)` 可以接受三个参数,分别表示序列的结束值(不包括)、开始值(默认为0)以及步长(默认为1)。例如:
```python
# 生成从0到4的序列(不包括5)
range(5)
# 输出:[0, 1, 2, 3]
# 生成从1到10,步长为2的序列
range(1, 11, 2)
# 输出:[1, 3, 5, 7, 9]
```
**numpy.arange() 函数**:
这是NumPy库中的函数,它类似于`range()`,但它可以生成浮点数序列,并且能够处理更大的数组范围,特别是对于大型计算任务非常有用。它的语法与`range()`类似,例如:
```python
import numpy as np
# 生成从0到4的等差浮点数序列(不包括5)
np.arange(5)
# 输出:array([0., 1., 2., 3., 4.])
# 生成从1到10,步长为0.5的浮点数序列
np.arange(1, 11, 0.5)
# 输出:array([1. , 1.5, 2. , 2.5, ..., 9.5, 10.])
```
相关问题
python arange
`arange()` 是一个 NumPy 库中的函数,用于创建一个数组,其中包含一个等间隔序列的值。它的语法如下:
```python
numpy.arange([start, ]stop, [step, ]dtype=None)
```
参数解释:
- `start`:可选,起始值,默认为 0;
- `stop`:必须,终止值(不包括),生成的值不包括该值;
- `step`:可选,两个相邻值之间的间隔,默认为 1;
- `dtype`:可选,返回数组的数据类型。
示例:
```python
import numpy as np
# 生成一个从 0 到 9 的一维数组
a = np.arange(10)
print(a) # [0 1 2 3 4 5 6 7 8 9]
# 生成一个从 2 到 9,步长为 2 的一维数组
b = np.arange(2, 10, 2)
print(b) # [2 4 6 8]
```
python的arange
Python的arange函数是numpy模块中的一个函数,用于创建一个包含指定范围内元素的数组。它的返回结果是一个ndarray对象,可以按照一定的步长生成一系列的数字。与之相比,range函数是Python自带的函数,返回的是一个整数列表(在Python 2中)或者是一个range对象(在Python 3中)。需要注意的是,使用arange函数之前需要先导入numpy模块。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [python中 range 与 arange 的用法与区别:](https://blog.csdn.net/weixin_50486192/article/details/124393090)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *3* [python入门--常用的numpy函数:arange()](https://blog.csdn.net/qq_44753801/article/details/105929180)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
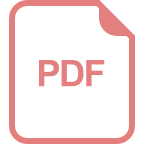
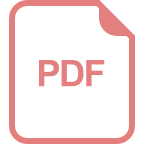
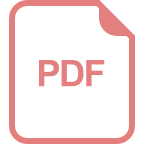












