整点报时数字钟multisimFPGA
时间: 2024-12-25 17:21:32 浏览: 17
### 设计思路
在Multisim中利用FPGA实现整点报时的数字钟电路涉及多个模块协同工作。该设计不仅需要精确的时间显示,还需要具备整点报时的功能[^4]。
### FPGA的选择与配置
对于此类应用,推荐选用支持VHDL或Verilog硬件描述语言编程的FPGA器件。通过这些高级抽象层次的语言编写程序来定义内部逻辑门阵列的行为模式,从而完成复杂功能的设计。Xilinx和Altera(现Intel)系列都是不错的选择,它们提供了丰富的开发工具链以及详尽的技术文档供开发者参考学习[^1]。
### 时间基准信号生成
采用NE555定时器构建多谐振荡器作为系统的时基发生源,产生周期稳定的方波脉冲序列供给后续各级处理单元使用。此部分负责提供整个系统运行所需的基础节拍——即每秒钟触发一次中断请求IRQ给CPU告知当前已过去一秒时间长度的信息。
```verilog
// Verilog code snippet for generating a 1Hz clock signal using NE555 timer simulation model.
module clk_gen_1hz (
input wire reset_n,
output reg clk_out
);
always @(posedge reset_n) begin
// Simplified representation of the actual implementation details here...
// In practice, this would involve configuring an external component like NE555 via appropriate interface signals.
clk_out <= ~clk_out;
end
endmodule
```
### 数字显示与时分秒计数
运用四位七段数码管LED显示器呈现直观可视化的实时时刻数据;同时借助于二进制编码十进制BCD(Binary-Coded Decimal)寄存器组保存并更新各个位上的数值变化情况。为了简化线路连接关系,在这里可以考虑引入专用集成电路IC74HC390来进行必要的预置加载、级联扩展等操作[^2]。
```vhdl
-- VHDL code snippet demonstrating BCD counter functionality with IC74HC390 equivalent behavior modeled within an FPGA fabric.
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity bcd_counter is
Port (
clk : in STD_LOGIC;
rst : in STD_LOGIC;
q : out STD_LOGIC_VECTOR(3 downto 0));
end entity;
architecture Behavioral of bcd_counter is
signal count_reg : unsigned(q'range);
begin
process(clk,rst)
begin
if(rising_edge(clk))then
if(rst='1') then
count_reg<=(others=>'0');
elsif(count_reg=9)then
count_reg<=to_unsigned(0,count_reg'length);
else
count_reg<=count_reg+1;
end if;
end if;
q<=std_logic_vector(count_reg);
end process;
end architecture ;
```
### 整点报时机制
当检测到分钟计数达到六十而秒针刚好指向零的位置时,则激活内置扬声器发出特定频率的声音提示用户新的一小时开始了。具体来说就是在上述提到过的主循环里加入额外的状态机判断条件用来识别这种情况的发生,并据此调用相应的音频播放子程序执行发声动作[^3]。
```c++
/* C++ pseudocode illustrating state machine logic behind hourly chime feature */
enum State {IDLE, CHIME_LOW, PAUSE_BETWEEN_CHIMES, CHIME_HIGH};
State currentState = IDLE;
int lowChimesCountdown = 4; // Number of lower-pitched beeps before high one at each hour mark.
void updateHourlyChimeLogic(int minutes, int seconds){
switch(currentState){
case IDLE:
if(minutes==0 && seconds==0){currentState=CHIME_LOW;}
break;
case CHIME_LOW:
playSound(LowFrequencyTone);
if(--lowChimesCountdown>0){currentState=PAUSE_BETWEEN_CHIMES;}else{currentState=CHIME_HIGH;}
break;
case PAUSE_BETWEEN_CHIMES:
delay(PauseDurationBetweenBeeps);
currentState=(lowChimesCountdown>0)?CHIME_LOW:CHIME_HIGH;
break;
case CHIME_HIGH:
playSound(HighFrequencyTone);
currentState=IDLE;
lowChimesCountdown=4;// Reset countdown for next occurrence
default:
break;
}
}
```
阅读全文
相关推荐


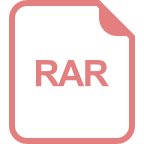



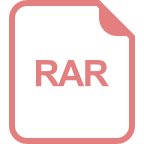





