数据结构C语言利用BF算法进行病毒检测的简单入门代码
时间: 2024-11-13 21:28:00 浏览: 9
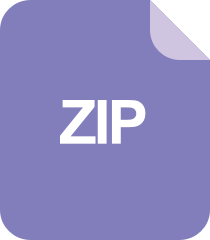
数据结构与算法的C语言课程代码.zip
在C语言中,我们可以使用Boyer-Moore (BM) 算法进行简单的字符串匹配,但这并不是直接用于病毒检测的。因为病毒检测通常涉及到复杂的文件分析和特征识别,需要更专业的病毒扫描引擎。然而,如果你想了解如何在C语言中运用 BM 算法,它主要用于查找特定模式在文本串中是否存在,这里是一个基本的示例:
```c
#include <stdio.h>
#include <string.h>
// 定义 Boyer-Moore 字符移动函数
int bad_char_shift(char target, char* pattern)
{
for (int i = 0; pattern[i] != '\0'; ++i)
if (pattern[i] == target)
return strlen(pattern) - 1;
return 0;
}
void boyer_moore_search(char* text, char* pattern)
{
int n = strlen(text);
int m = strlen(pattern);
// 将 pattern 中字符的位置反向存储
int shift[m];
for (int i = 0; i < m; ++i)
shift[i] = m - 1 - i;
int rightmost = n;
while (rightmost >= m)
{
bool match = 1;
for (int j = 0; j < m; ++j)
if (text[rightmost - j] != pattern[j])
{
match = 0;
break;
}
if (match)
printf("Pattern found at position %d\n", rightmost - m + 1);
// 更新移动位移
rightmost -= max(shift[text[rightmost]], 1);
}
}
int main()
{
char text[] = "Hello, world!";
char pattern[] = "world";
boyer_moore_search(text, pattern);
return 0;
}
```
这个代码展示了如何使用Boyer-Moore算法在一个字符串(`text`)中搜索另一个字符串(`pattern`)。它并不适用于病毒检测,但对于演示基本的字符串搜索算法是合适的。
阅读全文
相关推荐
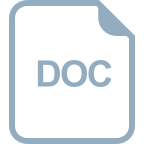
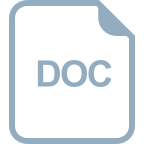
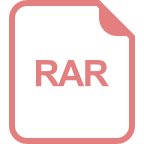
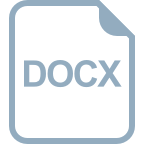
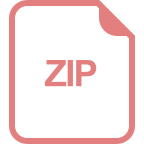
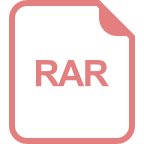
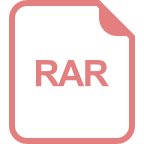
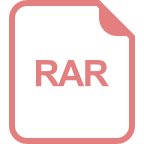
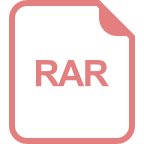
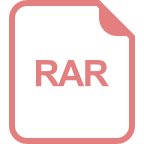
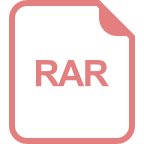
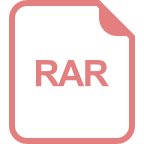
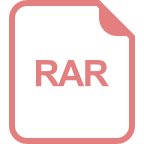
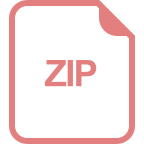