图片里面有个椭圆形水表将其用labelimg贴边标注,让图片旋转后,标的框仍能紧贴椭圆的边的代码
时间: 2024-12-23 18:15:47 浏览: 9
在使用LabelImg进行图像标注并保证图片旋转后标签框依然紧贴椭圆形边缘的过程中,你需要结合OpenCV、PIL等库来处理图像变换。这里是一个简单的Python代码示例:
```python
import cv2
from PIL import Image, ImageDraw
# 假设你已经保存了原始图片和对应的LabelImg标注文件
image_path = 'image.jpg'
mask_path = 'labels.png'
# 加载图片和标签
img = Image.open(image_path)
mask = Image.open(mask_path)
# 获取标签中的椭圆形边界坐标,这通常由LabelImg提供一个包含每个矩形信息的JSON文件,例如:
# {'boxes': [[x1, y1, x2, y2]], 'class_labels': ['elliptical_meter']...}
with open('mask_annotations.json', 'r') as f:
annotations = json.load(f)
box = annotations['boxes'][0]
# 椭圆的中心点和宽度、高度
center = (box[0], box[1])
width_height_ratio = (box[2] - box[0]) / (box[3] - box[1])
# 计算旋转后的椭圆坐标
def rotate_around_center(image, angle):
center_point = tuple(np.array(image.size) / 2)
rot_mat = cv2.getRotationMatrix2D(center_point, angle, 1.0)
return cv2.warpAffine(image, rot_mat, image.shape[::-1], borderMode=cv2.BORDER_CONSTANT)
angle = 45 # 你可以根据需求调整旋转角度
rotated_img = rotate_around_center(img, angle)
rotated_mask = rotate_around_center(mask, angle)
# 更新标签框,使其保持与旋转后的椭圆相对应
new_box = get_transformed_rectangle(box, angle, width_height_ratio)
rotated_mask_draw = ImageDraw.Draw(rotated_mask)
rotated_mask_draw.rectangle([new_box[0], new_box[1], new_box[2], new_box[3]], fill='white')
# 可以将新的图片和标注保存下来
rotated_img.save('rotated_image.jpg')
rotated_mask.save('rotated_labels.png')
def get_transformed_rectangle(rect, angle, ratio):
# 简单的假设椭圆长轴与X轴平行,计算旋转后的新矩形
new_rect = [rect[0], rect[1], rect[0] + ratio * (rect[2] - rect[0]), rect[1]]
matrix = cv2.getRotationMatrix2D((rect[0], rect[1]), angle, 1)
rotated_rect = np.dot(matrix, np.array(new_rect).reshape(2, 2)).flatten().tolist()
return rotated_rect
阅读全文
相关推荐





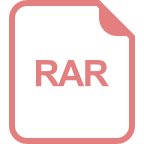











