使用Java向服务器上传文件压缩包并实现解压缩
时间: 2024-12-23 18:25:32 浏览: 4
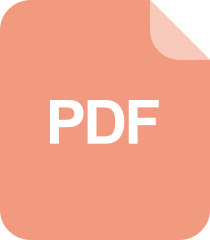
利用Java实现zip压缩/解压缩
在Java中,我们可以使用`java.util.zip`包和`java.io`包来上传文件和进行解压缩操作。以下是一个简单的示例,展示了如何上传压缩包,然后在服务器上解压:
1. **上传文件**:
首先,你需要一个网络连接到服务器。可以使用`java.net.URL`和`java.nio.file.Files`来发送文件。例如,使用`Files.copy()`方法从本地文件复制到服务器:
```java
URL serverUrl = new URL("http://your-server.com/upload");
File fileToUpload = new File("local-file.zip");
try (OutputStream out = serverUrl.openStream()) {
Files.copy(fileToUpload.toPath(), out);
} catch (IOException e) {
// handle exception
}
```
2. **上传压缩包**:
使用`multipart/form-data`形式上传,如果你使用了Spring MVC,可以在Controller里处理这样的请求:
```java
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file) {
try {
if (!file.isEmpty()) { // 检查是否为空
byte[] bytes = file.getBytes(); // 获取文件字节数组
sendBytesToServer(bytes); // 发送字节到服务器,这里需要自定义的发送方法
}
return "success";
} catch (Exception e) {
// handle error
}
return "error";
}
```
3. **解压缩文件**:
服务器接收到文件后,可以将其下载到内存或临时目录,然后使用`java.util.zip.ZipInputStream`来解压缩:
```java
ZipInputStream zipIn = null;
try {
zipIn = new ZipInputStream(new ByteArrayInputStream(bytes));
ZipEntry entry;
while ((entry = zipIn.getNextEntry()) != null) {
File destFile = new File("destination/" + entry.getName());
// 创建目录结构
if (!destFile.getParentFile().exists()) {
destFile.getParentFile().mkdirs();
}
FileOutputStream fos = new FileOutputStream(destFile);
byte[] buffer = new byte[1024];
int length;
while ((length = zipIn.read(buffer)) > 0) {
fos.write(buffer, 0, length);
}
fos.close();
}
zipIn.closeEntry();
} finally {
if (zipIn != null) {
try {
zipIn.close();
} catch (IOException e) {
// ignore
}
}
}
```
请注意,这个示例假设服务器有权限写入到指定的目录,而且对于大型文件或频繁操作,应该考虑使用更高效的文件I/O方法或库。
阅读全文
相关推荐
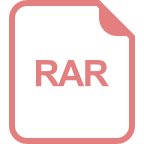
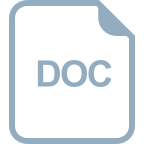
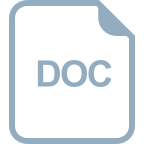
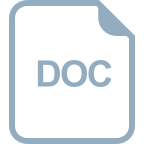
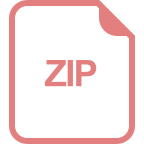
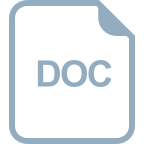
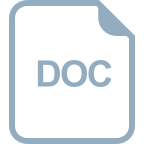
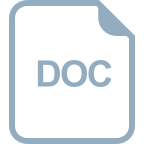
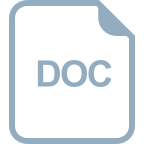
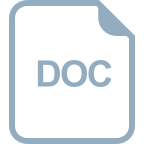
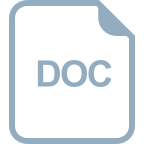
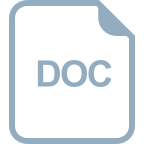
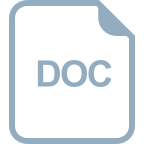
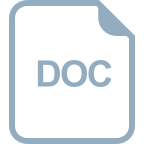
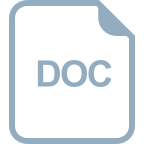
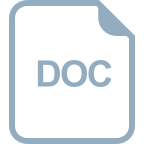
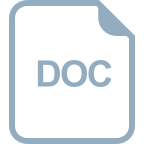
