利用cryptography库使用AES加密算法对数据进行加密和解密的具体程序300行长代码
时间: 2024-09-18 10:13:37 浏览: 76
在Python中,我们可以使用`cryptography`库来实现AES(Advanced Encryption Standard)加密和解密。下面是一个示例程序,展示如何创建一个简单的AES加密器和解密器:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
from cryptography.hazmat.backends import default_backend
import base64
# 导入密码和盐
password = b'secret_password'
salt = b'salt_example'
# 使用PBKDF2HMAC生成key和iv(初始化向量)
kdf = PBKDF2HMAC(
algorithm=algorithms.AES(),
length=32,
salt=salt,
iterations=100000,
backend=default_backend()
)
key = kdf.derive(password)
# 加密函数
def encrypt(data):
cipher = Cipher(algorithms.AES(key), modes.CBC(key[:16]), backend=default_backend())
encryptor = cipher.encryptor()
iv = key[16:]
ciphertext = encryptor.update(data) + encryptor.finalize()
return base64.b64encode(iv + ciphertext).decode('utf-8')
# 解密函数
def decrypt(encrypted_data):
encrypted_data = base64.b64decode(encrypted_data)
iv = encrypted_data[:16]
ciphertext = encrypted_data[16:]
cipher = Cipher(algorithms.AES(key), modes.CBC(iv), backend=default_backend())
decryptor = cipher.decryptor()
decrypted_data = decryptor.update(ciphertext) + decryptor.finalize()
return decrypted_data
# 示例:加密字符串
plaintext = 'This is a secret message'
encrypted_text = encrypt(plaintext.encode('utf-8'))
print(f"Encrypted data: {encrypted_text}")
# 示例:解密字符串
decrypted_text = decrypt(encrypted_text)
print(f"Decrypted data: {decrypted_text.decode('utf-8')}")
阅读全文
相关推荐




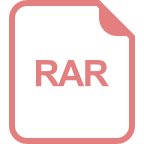










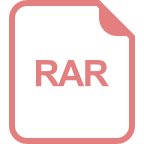
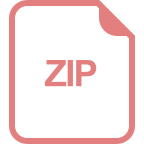