java crud 案例
时间: 2023-08-01 12:00:36 浏览: 51
Java CRUD案例指的是使用Java编程语言实现CRUD(Create, Read, Update, Delete)功能的示例。CRUD是指对于数据库中的数据实现基本的增删改查操作。
例如,假设有一个实体类Student,包含属性id、name、age,我们可以通过Java CRUD实现对该实体类的操作。
首先,我们可以定义一个Student类,该类包含私有属性id、name、age,并提供对应的getter和setter方法。
接下来,我们需要设计一个用于对Student类进行操作的数据库表,例如student_table,包含字段id、name、age。
对于CRUD的四个操作:
1. Create:创建操作可以通过在student_table中插入一条新的记录来实现,可以使用Java的JDBC(Java Database Connectivity)库来连接数据库,并执行插入操作。
2. Read:读取操作可以通过在student_table中查询记录来实现,可以使用JDBC库中的查询语句来获得满足条件的记录。
3. Update:更新操作可以通过更新student_table中的记录来实现,可以使用JDBC库中的更新语句来修改满足条件的记录。
4. Delete:删除操作可以通过删除student_table中的记录来实现,可以使用JDBC库中的删除语句来删除满足条件的记录。
通过Java CRUD案例,我们可以使用Java编程语言来实现对数据库中数据的增删改查操作,提供了一种方便灵活的方式来与数据库进行交互。
相关问题
javaspring项目完整案例
以下是一个简单的 Java Spring 项目案例,实现了用户注册、登录和资料修改功能:
1. 首先,你需要创建一个新的 Maven 项目。在 pom.xml 文件中添加以下依赖:
```xml
<dependencies>
<!-- Spring Framework -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.9</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>5.3.9</version>
</dependency>
<!-- Spring Security -->
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-web</artifactId>
<version>5.5.1</version>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-config</artifactId>
<version>5.5.1</version>
</dependency>
<!-- Thymeleaf -->
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf-spring5</artifactId>
<version>3.0.12.RELEASE</version>
</dependency>
<!-- MySQL数据库 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
<!-- Servlet API -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
</dependencies>
```
2. 创建数据库表
创建一个名为 `users` 的表,用来存储用户信息。表结构如下:
```
CREATE TABLE `users` (
`id` bigint(20) NOT NULL AUTO_INCREMENT,
`username` varchar(255) NOT NULL,
`password` varchar(255) NOT NULL,
`email` varchar(255) NOT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `username` (`username`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
3. 创建实体类
创建一个名为 `User` 的实体类,用来映射 `users` 表。
```java
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, unique = true)
private String username;
@Column(nullable = false)
private String password;
@Column(nullable = false)
private String email;
// 省略 getter 和 setter
}
```
4. 创建 DAO 接口
创建一个名为 `UserRepository` 的接口,继承 `JpaRepository` 接口,用来对 `users` 表进行 CRUD 操作。
```java
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
```
5. 创建 Service 接口和实现类
创建一个名为 `UserService` 的接口,定义注册、登录和资料修改等方法。
```java
public interface UserService {
void register(User user);
UserDetails login(String username, String password);
void updateProfile(User user);
}
```
创建一个名为 `UserServiceImpl` 的实现类,实现 `UserService` 接口。
```java
@Service
public class UserServiceImpl implements UserService, UserDetailsService {
@Autowired
private UserRepository userRepository;
@Autowired
private PasswordEncoder passwordEncoder;
@Override
public void register(User user) {
user.setPassword(passwordEncoder.encode(user.getPassword()));
userRepository.save(user);
}
@Override
public UserDetails login(String username, String password) {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
if (!passwordEncoder.matches(password, user.getPassword())) {
throw new BadCredentialsException("Invalid username or password");
}
return new UserPrincipal(user);
}
@Override
public void updateProfile(User user) {
userRepository.save(user);
}
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
return new UserPrincipal(user);
}
}
```
6. 创建控制器
创建一个名为 `UserController` 的控制器,用来处理用户注册、登录和资料修改等请求。
```java
@Controller
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/register")
public String showRegistrationForm(Model model) {
model.addAttribute("user", new User());
return "register";
}
@PostMapping("/register")
public String register(@ModelAttribute("user") @Valid User user, BindingResult result) {
if (result.hasErrors()) {
return "register";
}
userService.register(user);
return "redirect:/login";
}
@GetMapping("/login")
public String showLoginForm() {
return "login";
}
@PostMapping("/login")
public String login(@RequestParam String username, @RequestParam String password) {
userService.login(username, password);
return "redirect:/profile";
}
@GetMapping("/profile")
public String showProfileForm(Authentication authentication, Model model) {
User user = ((UserPrincipal) authentication.getPrincipal()).getUser();
model.addAttribute("user", user);
return "profile";
}
@PostMapping("/profile")
public String updateProfile(@ModelAttribute("user") @Valid User user, BindingResult result) {
if (result.hasErrors()) {
return "profile";
}
userService.updateProfile(user);
return "redirect:/profile";
}
}
```
7. 创建安全配置类
创建一个名为 `WebSecurityConfig` 的类,用来配置 Spring Security。
```java
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserService userService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/register", "/login").permitAll()
.antMatchers("/profile").authenticated()
.and()
.formLogin()
.loginPage("/login")
.defaultSuccessUrl("/profile")
.and()
.logout()
.logoutSuccessUrl("/login")
.and()
.csrf()
.disable();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userService).passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
8. 创建 Thymeleaf 模板
在 `src/main/resources/templates` 目录下创建以下模板文件:
register.html
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Register</title>
</head>
<body>
<h1>Register</h1>
<form th:action="@{/register}" th:object="${user}" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" th:field="*{username}" required>
<span th:if="${#fields.hasErrors('username')}" th:errors="*{username}"></span>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" th:field="*{password}" required>
<span th:if="${#fields.hasErrors('password')}" th:errors="*{password}"></span>
</div>
<div>
<label for="email">Email:</label>
<input type="email" id="email" th:field="*{email}" required>
<span th:if="${#fields.hasErrors('email')}" th:errors="*{email}"></span>
</div>
<div>
<button type="submit">Register</button>
</div>
</form>
</body>
</html>
```
login.html
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<form th:action="@{/login}" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
</div>
<div>
<button type="submit">Login</button>
</div>
</form>
</body>
</html>
```
profile.html
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Profile</title>
</head>
<body>
<h1>Profile</h1>
<form th:action="@{/profile}" th:object="${user}" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" th:field="*{username}" required>
<span th:if="${#fields.hasErrors('username')}" th:errors="*{username}"></span>
</div>
<div>
<label for="email">Email:</label>
<input type="email" id="email" th:field="*{email}" required>
<span th:if="${#fields.hasErrors('email')}" th:errors="*{email}"></span>
</div>
<div>
<button type="submit">Save</button>
</div>
</form>
</body>
</html>
```
9. 运行项目
运行项目,访问 `http://localhost:8080/register` 注册用户,然后访问 `http://localhost:8080/login` 登录,最后访问 `http://localhost:8080/profile` 修改个人资料。
java使用MongoDB实现存储客服聊天案例
可以使用MongoDB的Java驱动程序来实现存储客服聊天案例。首先,需要在Java应用程序中引入MongoDB的Java驱动程序。然后,使用MongoDB的Java驱动程序创建一个MongoClient实例,使用该实例连接到MongoDB服务器。接着,选择要使用的数据库和集合,并通过定义POJO类来映射存储在MongoDB中的数据。最后,可以使用MongoDB的Java驱动程序执行CRUD操作,从而实现存储和读取客服聊天数据。
相关推荐
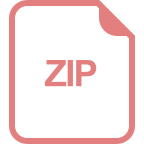












