c语言给定一个字符串87654321987654321求该字符串中各个数的个数
时间: 2024-10-19 13:08:01 浏览: 36
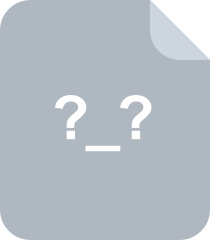
C语言程序设计-求一个给定字符串中的字母的个数.c

在C语言中,你可以通过遍历字符串并检查每个字符来计算数字的个数。这里是一个简单的示例程序:
```c
#include <stdio.h>
#include <ctype.h>
int count_digits(char str[]) {
int count = 0;
for(int i = 0; str[i] != '\0'; i++) { // 遍历字符串直到结束
if(isdigit(str[i])) { // 检查字符是否为数字
count++; // 数字个数加一
}
}
return count;
}
int main() {
char input[] = "87654321987654321";
int digit_count = count_digits(input);
printf("字符串 \"%s\" 中数字的个数是: %d\n", input, digit_count);
return 0;
}
```
这个程序会输出 "字符串 \"87654321987654321\" 中数字的个数是: 14"。
阅读全文
相关推荐
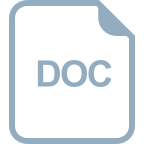















