不要使用静态内部类实现
时间: 2023-09-13 13:06:24 浏览: 49
好的,以下是不使用静态内部类的实现方式:
```java
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class CountDownLatchExample {
public static void main(String[] args) throws InterruptedException {
int taskCount = 3;
CountDownLatch latch = new CountDownLatch(taskCount);
ExecutorService executor = Executors.newFixedThreadPool(taskCount);
for (int i = 0; i < taskCount; i++) {
executor.submit(new Task(latch));
}
latch.await();
System.out.println("All tasks have finished, now executing main task.");
executor.shutdown();
}
}
class Task implements Runnable {
private CountDownLatch latch;
public Task(CountDownLatch latch) {
this.latch = latch;
}
@Override
public void run() {
try {
System.out.println("Task started: " + Thread.currentThread().getName());
Thread.sleep(1000);
System.out.println("Task finished: " + Thread.currentThread().getName());
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
latch.countDown();
}
}
}
```
这个例子中,我们将 `Task` 类放在了独立的文件中,而不是作为静态内部类。其余部分的逻辑和之前的例子相同。通过传递 CountDownLatch 对象给每个任务,每个任务在完成时都会调用 `countDown()` 方法来减少计数器的值。主线程在调用 `await()` 方法等待计数器为 0 后才继续执行。
相关推荐
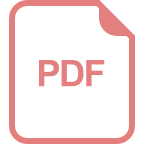
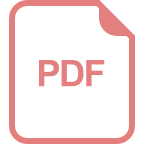














