输入一个正整数n,用高精度乘法求出阶乘和s,输入格式 一个正整数。 输出格式 计算结果。 C++
时间: 2024-10-03 13:01:36 浏览: 30
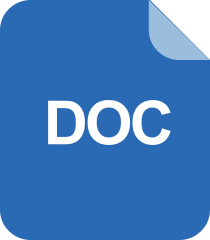
用Stirling逼近近似计算阶乘n!
在C++中,你可以使用`#include <iostream>`、`#include <string>`以及`#include <vector>`头文件,其中`<vector>`用于处理大整数,因为标准库的`std::integer_sequence`无法直接支持高精度乘法。下面是一个简单的示例,展示了如何使用`long long`类型和字符串来实现高精度乘法和阶乘计算:
```cpp
#include <iostream>
#include <string>
// 高精度乘法函数,将两个字符串转换成长整型相乘
std::string multiply(const std::string &a, const std::string &b) {
int lenA = a.size(), lenB = b.size();
std::vector<int> res(lenA + lenB + 1);
for (int i = lenA - 1; i >= 0; --i) {
for (int j = lenB - 1; j >= 0; --j) {
int mul = a[i] - '0' * 10 + b[j] - '0' * 10;
res[lenA + j - i - 1] += mul;
if (res[lenA + j - i - 1] >= 10) {
res[lenA + j - i - 1] -= 10;
}
}
}
// 将结果从左向右填充零直到合适长度
while (res[0] == 0 && res.size() > 1) {
res.erase(res.begin());
}
return std::to_string(res.back()) + std::string(res.begin(), res.end() - 1);
}
// 计算阶乘
std::string factorial(const std::string &n) {
if (n == "1") return "1";
std::string fact = multiply(n, "1");
for (size_t i = 2; i < n.size(); ++i) {
fact = multiply(fact, n.substr(0, i));
}
return fact;
}
int main() {
int n;
std::cin >> n;
std::string input_num = std::to_string(n);
std::string result = factorial(input_num);
std::cout << "计算结果: " << result << std::endl;
return 0;
}
```
阅读全文
相关推荐
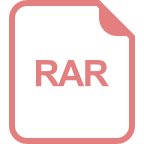
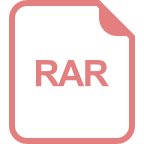

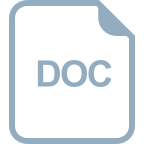
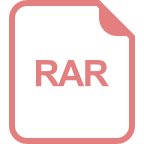
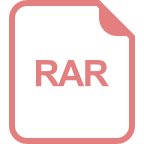
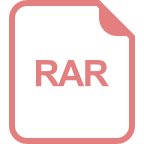
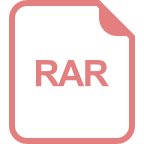
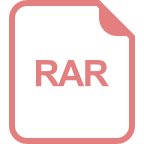
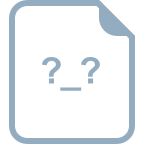
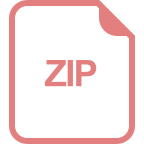
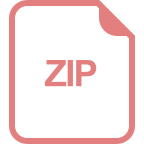
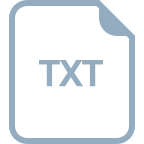
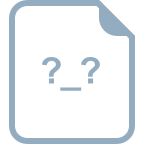
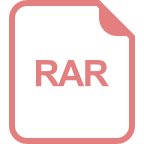
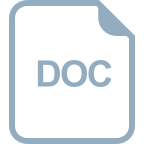
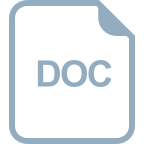