用python求解两空间直线交点
时间: 2024-02-28 12:50:23 浏览: 158
在Python中,可以使用向量和线性代数的知识来求解两个空间直线的交点。下面是一个求解两个三维空间直线交点的示例代码:
```python
import numpy as np
# 定义两个直线的参数方程
def line1(t):
x = 2 + 3*t
y = 1 - t
z = 4 + 2*t
return np.array([x, y, z])
def line2(s):
x = 1 + s
y = 2 - 2*s
z = 3 + 3*s
return np.array([x, y, z])
# 求解交点
def find_intersection():
# 构造直线的参数方程表示
A = np.array([line1(1) - line1(0), line2(1) - line2(0)]).T
b = line2(0) - line1(0)
# 使用线性代数库求解方程组
try:
t, s = np.linalg.solve(A, b)
intersection_point = line1(t)
return intersection_point
except np.linalg.LinAlgError:
# 方程组无解,直线平行或重合
return None
# 调用函数求解交点
intersection = find_intersection()
if intersection is not None:
print("两直线的交点为:", intersection)
else:
print("两直线平行或重合,无交点")
```
这段代码中,我们首先定义了两个直线的参数方程`line1`和`line2`,然后构造了一个方程组,通过线性代数库中的`np.linalg.solve`函数求解方程组,得到交点的参数`t`和`s`,最后通过参数方程计算出交点的坐标。如果方程组无解,则说明两条直线平行或重合,无交点。
阅读全文
相关推荐
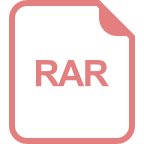
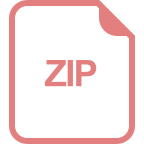
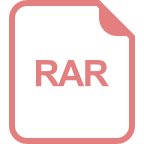
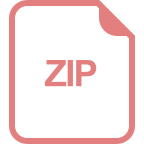
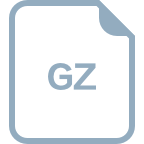
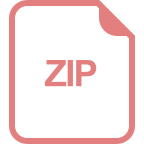
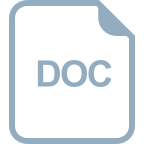
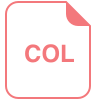
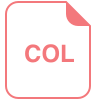
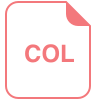
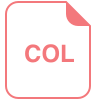
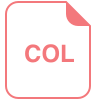
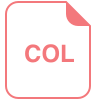
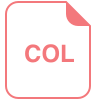
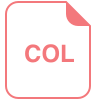

