HashMap和ConcurrentHashMap有什么区别?
时间: 2024-06-01 18:07:01 浏览: 115
HashMap 和 ConcurrentHashMap 都是 Java 中常用的哈希表实现,它们之间的主要区别如下:
- 并发性:ConcurrentHashMap 支持并发读写操作,而 HashMap 不支持并发操作。
- 线程安全:ConcurrentHashMap 是线程安全的,而 HashMap 不是线程安全的。
- 锁机制:ConcurrentHashMap 使用分段锁(Segment),每一个 Segment 相当于一个小的 HashMap,只锁住当前需要操作的部分,而 HashMap 使用全局锁 synchronized。
- 效率:在高并发读写场景下,ConcurrentHashMap 的效率比 HashMap 更高。但是在单线程下,HashMap 的效率要比 ConcurrentHashMap 更高。
下面是一个使用 HashMap 和 ConcurrentHashMap 的例子:
```java
import java.util.HashMap;
import java.util.concurrent.ConcurrentHashMap;
public class MapExample {
public static void main(String[] args) {
// 使用 HashMap 实现
HashMap<String, Integer> hashMap = new HashMap<>();
hashMap.put("A", 1);
hashMap.put("B", 2);
hashMap.put("C", 3);
System.out.println(hashMap.get("A")); // 输出:1
// 使用 ConcurrentHashMap 实现
ConcurrentHashMap<String, Integer> concurrentHashMap = new ConcurrentHashMap<>();
concurrentHashMap.put("A", 1);
concurrentHashMap.put("B", 2);
concurrentHashMap.put("C", 3);
System.out.println(concurrentHashMap.get("A")); // 输出:1
}
}
```
阅读全文
相关推荐
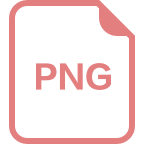
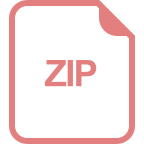
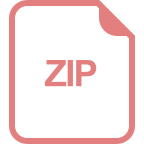
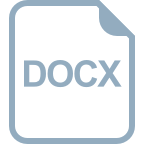
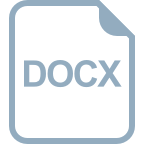
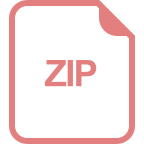