基于人工智能的交通违法行为识别系统代码实现
时间: 2024-10-29 12:19:42 浏览: 17
基于人工智能的交通违法行为识别系统通常会结合计算机视觉技术和深度学习算法,例如卷积神经网络(CNN)。以下是简化的代码实现步骤概述:
```python
# 导入必要的库
import cv2
import numpy as np
from tensorflow.keras.models import load_model
# 加载预训练的人脸检测模型(如MTCNN)
face_detector = cv2.dnn.readNetFromCaffe('deploy.prototxt', 'res10_300x300_ssd_iter_140000.caffemodel')
# 加载违法动作识别模型(如YOLO或Faster R-CNN)
action_recognition_model = load_model('traffic_rules.h5')
def identify_violation(image_path):
# 读取图像
img = cv2.imread(image_path)
# 预处理图像,调整大小并进行人脸检测
blob = cv2.dnn.blobFromImage(img, 1.0, (300, 300), [104, 177, 123])
face_detector.setInput(blob)
faces = face_detector.forward()
for detection in faces[0, 0, :, :]:
x1, y1, width, height = detection[3:7] * np.array([img.shape[1], img.shape[0], img.shape[1], img.shape[0]])
face_roi = img[y1:y1 + height, x1:x1 + width]
# 对面部区域进行裁剪并缩放到模型需要的尺寸
resized_face = cv2.resize(face_roi, (input_shape[1], input_shape[0]))
# 进行违法动作识别
features = preprocess_image(resized_face)
prediction = action_recognition_model.predict(features)
# 根据预测概率判断违法行为
if prediction.max() > threshold:
violation_type = get_action_label(np.argmax(prediction))
print(f"Detected violation: {violation_type}")
return img
# 其他辅助函数...
```
这个例子中,假设已经有了预训练好的人脸检测和违法行为分类模型。实际部署时,你需要准备相应的模型文件、数据预处理函数(`preprocess_image`)以及将预测类别映射回真实违法行为名称的函数(`get_action_label`)。
阅读全文
相关推荐
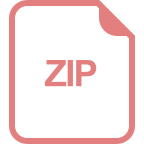
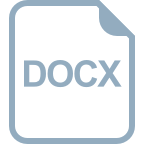
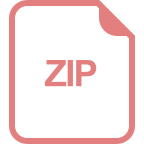
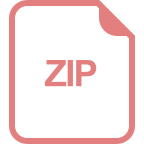
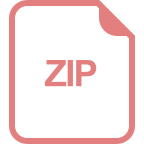
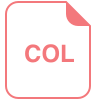
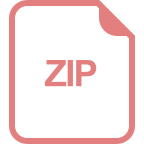
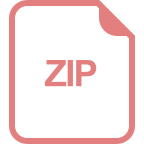
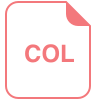
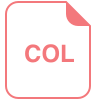
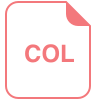
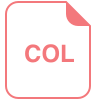
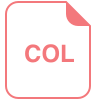
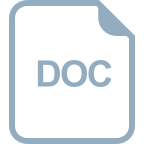
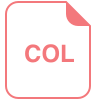
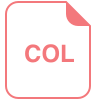
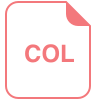
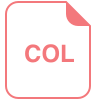
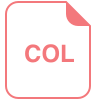