html中href引入css文件,文件后面拼一个变量
时间: 2024-10-23 21:20:48 浏览: 11
在HTML中,`<link>` 标签通常用于引入外部CSS文件,通过`href`属性指定样式表的位置。如果你想动态地拼接变量来引用CSS文件,你可以这样做:
```html
<link rel="stylesheet" type="text/css" href="styles.css?id=<%= yourVariable %>" />
```
这里假设`yourVariable`是一个服务器端脚本(如JavaScript、PHP、ASP.NET等)生成的变量,并将其值替换到URL中。例如,在JavaScript中,你可以像下面这样设置变量:
```javascript
var yourVariable = "custom-style";
document.querySelector('link[href]').setAttribute('href', 'styles.css?id=' + yourVariable);
```
这将把当前页面的`<link>`元素的`href`指向`styles.css?id=custom-style`。注意,这种方式需要服务器端支持动态内容渲染,因为浏览器本身并不具备解析动态变量的能力。
相关问题
href引入css文件,文件后面拼一个动态变量
当你需要在HTML中通过href属性引入CSS文件,并希望该文件名包含动态内容时,你可以使用字符串模板或者JavaScript将动态变量插入到URL路径中。例如:
```html
<!-- 使用JavaScript动态插入变量 -->
<script>
var dynamicVariable = "styles_"; // 动态变量,假设它代表一个ID或版本号
var cssFileUrl = 'styles.css' + dynamicVariable; // 将变量添加到静态路径后
document.head.appendChild(
document.createElement('link')
.setAttribute('rel', 'stylesheet')
.setAttribute('href', cssFileUrl)
);
</script>
<!-- 或者使用模板字符串 -->
<link rel="stylesheet" href={`styles.css${dynamicVariable}`}>
```
在这个例子中,`dynamicVariable`会被替换到最终的CSS文件URL中,如 `styles.cssstyles_`。这种方法允许你在运行时更改样式文件名,比如根据用户偏好、时间戳或是其他条件。
vue中根据条件动态引入css文件
在Vue中可以通过动态添加link标签的方式来引入CSS文件,具体实现如下:
1. 在组件中定义一个变量来存储CSS文件的路径,例如:
```
data() {
return {
cssPath: 'path/to/css/file.css',
}
}
```
2. 在组件的mounted生命周期钩子函数中,创建一个link标签并动态添加到页面中,例如:
```
mounted() {
const link = document.createElement('link')
link.rel = 'stylesheet'
link.href = this.cssPath
document.head.appendChild(link)
}
```
3. 如果需要根据条件动态引入不同的CSS文件,可以通过计算属性来实现,例如:
```
computed: {
cssPath() {
return this.condition ? 'path/to/css/file1.css' : 'path/to/css/file2.css'
}
}
```
其中,condition为条件判断语句,根据不同的条件返回不同的CSS文件路径。
需要注意的是,当组件销毁时,需要将动态添加的link标签从页面中移除,以避免影响其他组件的样式。可以在组件的beforeDestroy生命周期钩子函数中实现,例如:
```
beforeDestroy() {
const link = document.querySelector(`link[href="${this.cssPath}"]`)
link && link.parentNode.removeChild(link)
}
```
阅读全文
相关推荐
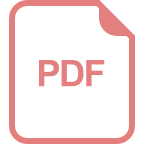
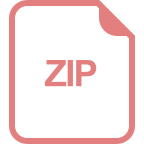
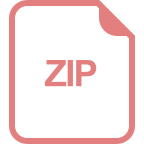
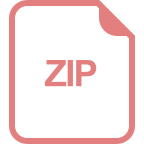
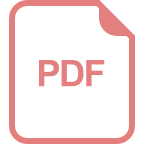
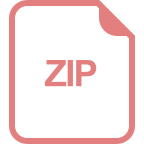
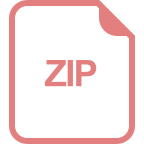
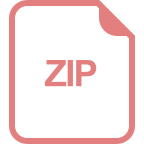
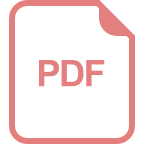
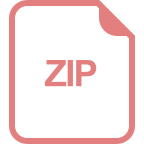
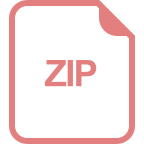
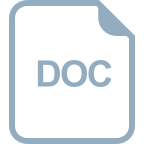
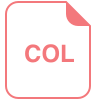


