c语言编写函数对结构体类型数据进行排序
时间: 2023-12-15 10:01:47 浏览: 317
C语言编写函数对结构体类型数据进行排序的方法有很多种。下面给出一种常见的实现方法。
首先,定义一个包含多个成员的结构体类型,例如学生信息结构体Student,其中包含成员name(姓名)、age(年龄)和score(分数)。
接下来,编写一个排序函数sort,该函数接受一个Student类型的数组和数组大小作为参数。在排序过程中,可以使用常见的排序算法,比如冒泡排序、快速排序、插入排序等。这里以冒泡排序为例演示排序过程。
```c
#include <stdio.h>
#include <string.h>
// 定义学生信息结构体类型
typedef struct {
char name[100];
int age;
float score;
} Student;
// 排序函数
void sort(Student arr[], int size) {
int i, j;
Student temp;
for(i = 0; i < size - 1; i++) {
for(j = 0; j < size - 1 - i; j++) {
// 使用strcmp函数比较字符串大小
if(strcmp(arr[j].name, arr[j + 1].name) > 0) {
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int main() {
Student students[] = {
{"Alice", 18, 89.5},
{"Bob", 20, 77.8},
{"Cathy", 19, 95.3}
};
int size = sizeof(students) / sizeof(students[0]);
sort(students, size);
for(int i = 0; i < size; i++) {
printf("name: %s, age: %d, score: %.1f\n", students[i].name, students[i].age, students[i].score);
}
return 0;
}
```
运行该程序,输出结果为:
```
name: Alice, age: 18, score: 89.5
name: Bob, age: 20, score: 77.8
name: Cathy, age: 19, score: 95.3
```
以上就是使用C语言编写函数对结构体类型数据进行排序的一个示例。通过定义自定义的结构体类型和使用合适的排序算法,可以实现对结构体类型数据的排序。
阅读全文
相关推荐








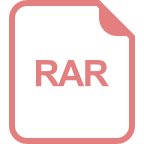


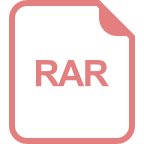

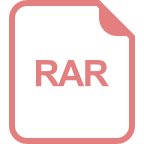




