用c++的类写一个一个班级的学生选课和查看各科成绩和排名
时间: 2024-10-16 11:03:27 浏览: 22
在C++中,我们可以创建一个名为`Class`的类来表示学生,然后添加`Course`类来表示课程,以及相关的属性如`name`、`scores`等。这里是一个简单的示例:
```cpp
#include <iostream>
#include <vector>
#include <map>
#include <algorithm>
class Course {
public:
std::string name;
int maxScore; // 设定每门课的最大分值
private:
std::vector<int> scores; // 用于存储每个学生的分数
};
class Student {
public:
std::string id;
std::map<Course*, int> courseScores; // 学生选课的成绩映射
// 选择课程并设置成绩
void selectCourse(Course* course, int score) {
if (courseScores.find(course) == courseScores.end()) {
courseScores[course] = score;
} else {
std::cout << "Student already selected this course!" << std::endl;
}
}
// 查看单门课程的成绩
int getScore(const Course* course) const {
auto it = courseScores.find(course);
if (it != courseScores.end()) {
return it->second;
} else {
throw std::runtime_error("Student has not taken this course.");
}
}
// 打印所有课程的成绩
void printScores() const {
for (const auto& pair : courseScores) {
std::cout << "Course: " << pair.first->name << ", Score: " << pair.second << std::endl;
}
}
// 按照某一门课程的成绩排序
bool operator<(const Student& other) const {
return getScore(&courseScores.begin()->first) < other.getScore(&courseScores.begin()->first);
}
};
int main() {
Course math{ "Math", 100 };
Course physics{ "Physics", 100 };
Student student1{ "001" };
student1.selectCourse(&math, 95);
student1.selectCourse(&physics, 90);
student1.printScores();
// 排序按数学成绩,这里只是一个演示,实际场景可以根据需要调整
std::vector<Student> students;
students.push_back(student1);
// ... 添加其他学生...
std::sort(students.begin(), students.end());
for (const auto& student : students) {
std::cout << "Student: " << student.id << " Rank: " << std::distance(students.begin(), std::find_if(students.begin(), students.end(), student)) + 1 << std::endl;
}
return 0;
}
```
在这个例子中,我们实现了学生选课、查看成绩、打印所有成绩,并按照一门特定课程的成绩对学生进行简单排序的功能。
阅读全文
相关推荐
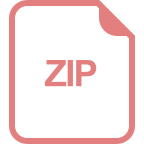
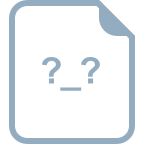
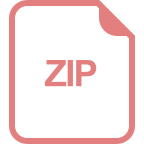


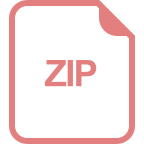
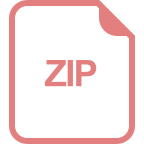
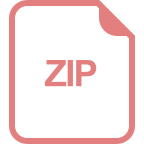
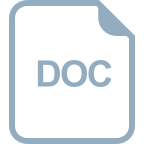
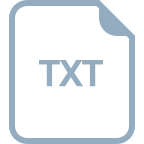
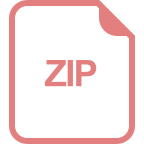
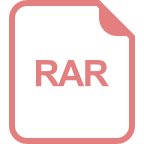
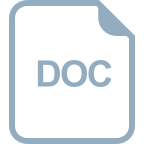
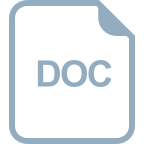



