python温度动态变化云图
时间: 2023-08-07 14:11:51 浏览: 222
要创建一个温度动态变化的云图,你可以使用Python的matplotlib库和OpenCV库。下面是一个简单的步骤:
1. 导入所需的库
```python
import matplotlib.pyplot as plt
import cv2
import numpy as np
import time
```
2. 创建一个函数来生成温度云图的每一帧
```python
def generate_frame():
# 根据当前的温度数据生成温度云图
# 返回一个RGB图像,表示温度云图的一帧
# 示例代码:使用随机数据生成一个伪造的温度云图
width, height = 640, 480
temperature_map = np.random.rand(height, width) * 100 # 生成0到100之间的随机温度数据
# 使用matplotlib绘制热图
plt.imshow(temperature_map, cmap='hot', interpolation='nearest')
plt.axis('off')
# 将热图转换为RGB格式
fig = plt.gcf()
fig.canvas.draw()
image = np.array(fig.canvas.renderer._renderer)
plt.close()
return cv2.cvtColor(image, cv2.COLOR_RGBA2BGR)
```
3. 创建一个视频文件并将温度云图的每一帧写入视频文件
```python
# 设置视频文件的属性
width, height = 640, 480
fps = 30.0
video_filename = 'temperature_cloud.mp4'
# 创建视频编写器对象
fourcc = cv2.VideoWriter_fourcc(*'mp4v')
out = cv2.VideoWriter(video_filename, fourcc, fps, (width, height))
# 设置动态变化的温度数据
total_frames = 100 # 总帧数
start_temperature = 20 # 起始温度
end_temperature = 80 # 终止温度
# 生成并写入每一帧
for i in range(total_frames):
# 计算当前帧的温度数据
current_temperature = start_temperature + (end_temperature - start_temperature) * i / total_frames
# 更新温度数据并生成帧
frame = generate_frame(current_temperature)
out.write(frame)
# 可选:添加延迟,以便观察每一帧的变化
time.sleep(0.1)
# 释放视频编写器对象
out.release()
```
请注意,上面的代码只是一个示例,用于生成一个伪造的温度云图,并将其保存为视频文件。你需要根据你的实际需求,使用适当的温度数据和生成温度云图的方法。另外,你还可以根据需要调整视频文件的属性,如宽度、高度和帧率,并在每一帧之间添加适当的延迟以控制动画速度。
阅读全文
相关推荐


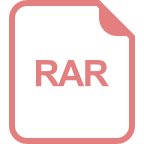
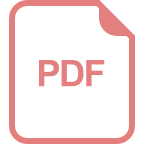
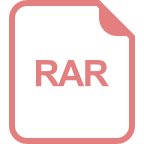
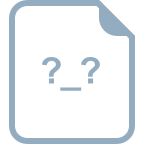
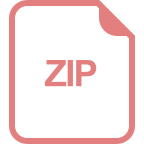
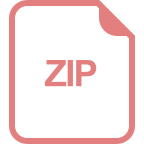
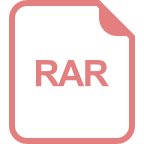
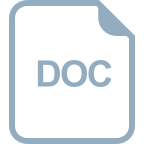
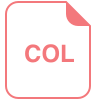


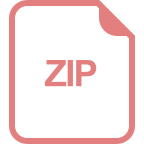