vue+ts将页面内容赋值并跳转到另一个页面
时间: 2024-09-11 17:02:49 浏览: 88
在Vue项目中使用TypeScript(TS)进行页面内容赋值并实现页面跳转,通常会涉及到以下几个步骤:
1. 定义数据源:首先需要在组件中定义你想要传递的数据,使用TypeScript进行类型声明,以保证数据的类型安全。
```typescript
import { Vue, Component } from 'vue-property-decorator';
@Component
export default class MyComponent extends Vue {
private myData: string; // 定义一个字符串类型的数据
mounted() {
this.myData = '要传递的数据'; // 在组件的某个生命周期钩子中赋值
}
}
```
2. 路由跳转:在Vue中通常使用vue-router进行页面跳转。你需要配置路由并设置好跳转逻辑。
```javascript
// router/index.ts
import Vue from 'vue';
import Router from 'vue-router';
import AnotherPage from '@/components/AnotherPage'; // 另一个页面的组件
Vue.use(Router);
export default new Router({
routes: [
{
path: '/anotherPage',
name: 'AnotherPage',
component: AnotherPage
},
// ...其他路由配置
]
});
```
3. 传递数据:使用vue-router的`router.push`方法进行页面跳转,并传递数据。
```typescript
// 假设在某个方法中需要进行跳转
import { Vue, Component } from 'vue-property-decorator';
import router from '@/router'; // 引入路由配置
@Component
export default class MyComponent extends Vue {
// ...其他代码
goToAnotherPage() {
this.$router.push({ name: 'AnotherPage', params: { data: this.myData } });
}
}
```
4. 接收数据:在目标页面组件中,通过路由对象`this.$route.params`接收传递过来的数据。
```typescript
import { Vue, Component } from 'vue-property-decorator';
import { Route } from 'vue-router';
@Component
export default class AnotherComponent extends Vue {
private receivedData: string; // 定义接收数据的属性
created() {
const route: Route = this.$route; // 获取当前路由信息
this.receivedData = route.params.data; // 从路由参数中获取传递的数据
}
}
```
完成以上步骤后,就可以在Vue+TS项目中实现页面内容的赋值并跳转到另一个页面了。
阅读全文
相关推荐
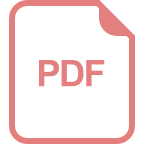
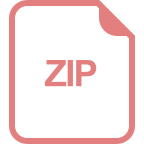
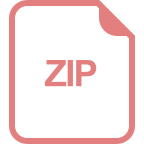


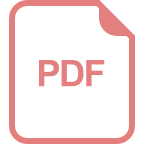
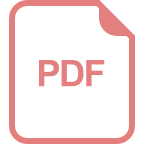
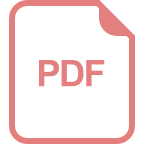
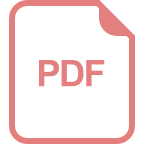
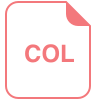






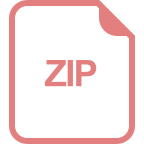
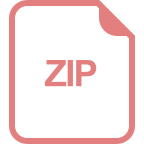
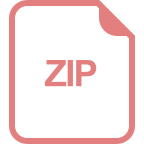