vscode在终端编译opebcv c++文件
时间: 2024-09-06 09:07:09 浏览: 118
Visual Studio Code(VSCode)是一款由微软开发的轻量级但功能强大的源代码编辑器,它支持多种编程语言的开发,包括C++。要使用VSCode在终端中编译OpenCV C++文件,您需要遵循以下步骤:
1. 确保您的系统上安装了C++编译器,如GCC或Clang,以及CMake(如果您的项目使用CMake构建)。
2. 安装OpenCV库并配置好环境变量,以便在项目中使用。
3. 在VSCode中打开您的C++文件所在的项目文件夹。
4. 安装C++扩展,如C/C++扩展(由Microsoft提供),这将提供语言支持和IntelliSense功能。
5. 创建一个构建脚本,例如`build.sh`(Linux/macOS)或`build.bat`(Windows),在脚本中编写编译命令,调用g++或cl.exe等编译器。编译命令通常包括编译器路径、源文件、头文件路径、库文件路径以及编译选项。
6. 在VSCode中打开终端(Terminal > New Terminal),并运行您创建的构建脚本。例如,如果您使用的是Linux系统,可以在终端中输入如下命令:
```
./build.sh
```
或者如果是Windows系统:
```
build.bat
```
7. 如果编译成功,您可以运行生成的可执行文件来测试程序。
相关问题
vscode 使用cmake配置opencv c++项目
要在VS Code中使用CMake配置OpenCV C++项目,可以按照以下步骤进行操作:
1. 确保已经安装了Visual Studio Code和CMake,并将它们添加到系统的环境变量中。
2. 创建一个新的文件夹作为您的项目文件夹,并在其中创建一个CMakeLists.txt文件。在CMakeLists.txt中,输入以下内容:
```cmake
cmake_minimum_required(VERSION 3.0)
project(YourProjectName)
find_package(OpenCV REQUIRED)
add_executable(YourExecutableName main.cpp)
target_link_libraries(YourExecutableName ${OpenCV_LIBS})
```
这个CMakeLists.txt文件指定了项目的最低CMake版本、项目名称以及要使用的OpenCV库。
3. 在项目文件夹中创建一个main.cpp文件,并编写您的OpenCV C++代码。
4. 打开Visual Studio Code,选择“文件”->“打开文件夹”,并选择您的项目文件夹。
5. 安装CMake Tools插件。在Visual Studio Code的扩展面板中搜索"CMake Tools"并安装。
6. 在Visual Studio Code的底部状态栏,找到一个齿轮图标,点击它以打开CMake Tools。
7. 在CMake Tools的侧边栏中,选择您的项目文件夹并点击“配置”。
8. 在弹出的对话框中,选择一个构建目录(可以是您项目文件夹下的子文件夹),然后选择CMake工具链。
9. 在弹出的对话框中,输入以下参数并保存:
- "cmake.configureOnOpen": false
- "cmake.buildDirectory": "build"
这些参数将配置CMake Tools以在打开项目时不自动运行CMake,并将生成的文件放在名为"build"的文件夹中。
10. 回到CMake Tools的侧边栏,点击“配置”按钮,然后选择“生成”按钮。这将运行CMake生成您的项目。
11. 在CMake Tools的侧边栏中,点击“构建”按钮来编译您的项目。
12. 您可以在Visual Studio Code的终端中运行您的可执行文件,或者根据需要进行调试。
这样,您就可以在VS Code中使用CMake配置OpenCV C++项目了。记得根据您的具体项目情况修改CMakeLists.txt和main.cpp文件。祝您成功!
vscode配置c++和opencv环境
要在VScode中配置C++和OpenCV环境,你可以按照以下步骤进行操作:
1. 首先,确保你已经安装了OpenCV库。你可以参考[1]中提供的链接来安装OpenCV。
2. 打开VScode,创建一个新的C++项目。
3. 在项目目录下放入一张图片,以便后续测试。
4. 创建一个名为`main.cpp`的文件,并将[2]中提供的代码复制粘贴到该文件中。
5. 打开VScode的终端,并执行以下命令来安装必要的依赖项:
```
sudo apt-get install build-essential
sudo apt-get install cmake
```
6. 在VScode中打开`tasks.json`文件,并将[3]中提供的配置代码复制粘贴到该文件中。
7. 保存并关闭`tasks.json`文件。
8. 在VScode中打开`launch.json`文件,并将以下配置代码复制粘贴到该文件中:
```
{
"version": "0.2.0",
"configurations": [
{
"name": "C++ Launch",
"type": "cppdbg",
"request": "launch",
"program": "${workspaceFolder}/a.out",
"args": [],
"stopAtEntry": false,
"cwd": "${workspaceFolder}",
"environment": [],
"externalConsole": false,
"MIMode": "gdb",
"miDebuggerPath": "/usr/bin/gdb"
}
]
}
```
9. 保存并关闭`launch.json`文件。
10. 现在你可以在VScode中编译和运行你的C++代码了。按下F5键或选择调试菜单中的"Start Debugging"选项即可开始调试。
请注意,以上步骤假设你的操作系统是Ubuntu,并且已经正确安装了OpenCV库。如果你的操作系统或安装方式不同,可能需要进行适当的调整。
希望这些步骤能帮助你成功配置C++和OpenCV环境。如果你有任何进一步的问题,请随时提问。
阅读全文
相关推荐
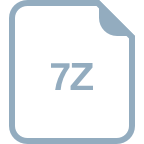
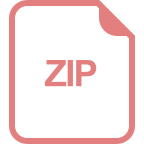
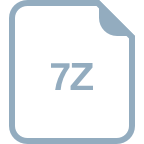
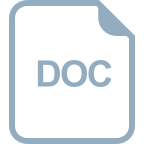
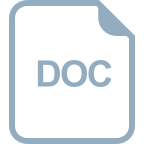
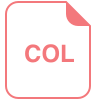









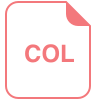
