vue父组件调用子组件的方法和给子组件的属性赋值
时间: 2023-11-23 16:06:46 浏览: 99
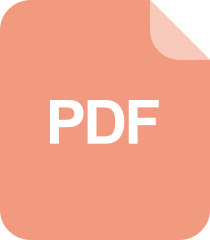
vue 父组件中调用子组件函数的方法

Vue中父组件调用子组件的方法和给子组件的属性赋值有以下几种方法:
1. 使用prop传值:在子组件中设置prop属性,然后在父组件中引入子组件并传递参数即可。这样父组件和子组件之间形成了一个单向下行绑定,父级prop的更新会向下流动到子组件中,但是反过来则不行。
2. 使用$emit方法传值:在子组件中使用$emit方法触发一个自定义事件,并将需要传递的参数作为$emit方法的第二个参数传递。在父组件中监听这个自定义事件,并在事件处理函数中获取传递的参数。
3. 使用$refs属性调用子组件的方法:在父组件中使用ref属性给子组件命名,然后通过$refs属性获取子组件实例,从而调用子组件的方法。
4. 使用$parent属性调用父组件的方法:在子组件中使用$parent属性获取父组件实例,从而调用父组件的方法。
注意,使用$parent属性调用父组件的方法可能会导致代码的可维护性变差,因此应该尽量避免使用。同时,使用$refs属性调用子组件的方法也应该谨慎使用,因为这样会使父组件和子组件之间产生耦合。
阅读全文
相关推荐
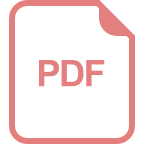
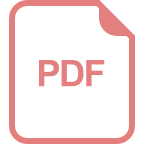
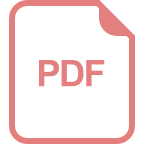
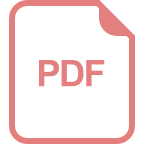
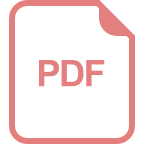
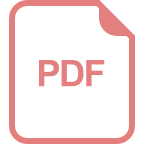
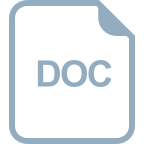









