linux管道符把一个文件里的传到另一个文件
时间: 2024-09-11 19:01:28 浏览: 40
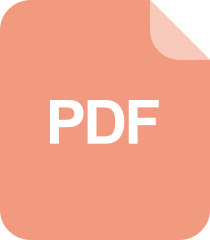
linux shell 管道命令(pipe)使用及与shell重定向区别
在Linux系统中,管道符通常用`|`表示,它可以将一个命令的标准输出作为另一个命令的标准输入。如果你想要将一个文件的内容传递给另一个文件,实际上你不需要使用管道符,因为管道符主要用于命令间的数据传递。你可以使用重定向操作符`>`来实现文件内容的复制。
例如,如果你想将一个名为`sourcefile.txt`的文件内容复制到另一个名为`destinationfile.txt`的文件中,你可以使用以下命令:
```bash
cat sourcefile.txt > destinationfile.txt
```
这里,`cat`命令用于读取文件内容,并通过`>`操作符将输出重定向到`destinationfile.txt`文件中。如果`destinationfile.txt`不存在,它会被创建;如果已存在,它会被覆盖。
如果你想合并多个文件的内容到一个文件中,可以使用`cat`命令结合文件名列表:
```bash
cat file1.txt file2.txt file3.txt > allfiles.txt
```
这会将`file1.txt`、`file2.txt`和`file3.txt`的内容合并后保存到`allfiles.txt`文件中。
阅读全文
相关推荐
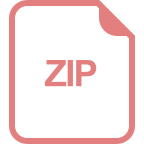
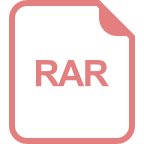















