凯撒密码加密解密算法nodejs
时间: 2023-10-18 15:05:44 浏览: 173
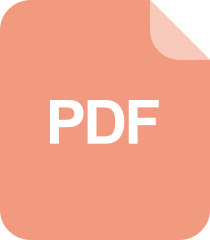
nodejs中各种加密算法的实现详解
凯撒密码是一种基于字母移位的代换密码,通过将字母移动一定的位数来进行加密和解密。在Node.js中,可以使用crypto模块中的Cipher类和Decipher类来实现凯撒密码的加密和解密操作。
首先,使用Cipher类进行加密操作。可以使用crypto模块的createCipher方法创建一个Cipher对象,并指定加密算法和密钥。然后,可以使用Cipher对象的update方法传入要加密的数据,并指定编码格式,最后使用final方法获取加密完成的数据。
例如,下面的代码演示了使用凯撒密码对明文进行加密:
```
const crypto = require('crypto');
function caesarCipherEncrypt(text, shift) {
const cipher = crypto.createCipher('aes192', 'secret_key');
let encrypted = cipher.update(text, 'utf8', 'hex');
encrypted += cipher.final('hex');
return encrypted;
}
const plaintext = 'Hello, World!';
const shift = 3;
const ciphertext = caesarCipherEncrypt(plaintext, shift);
console.log(ciphertext);
```
接下来,使用Decipher类进行解密操作。同样,可以使用crypto模块的createDecipher方法创建一个Decipher对象,并指定解密算法和密钥。然后,可以使用Decipher对象的update方法传入要解密的数据,并指定编码格式,最后使用final方法获取解密完成的数据。
例如,下面的代码演示了使用凯撒密码对密文进行解密:
```
function caesarCipherDecrypt(ciphertext, shift) {
const decipher = crypto.createDecipher('aes192', 'secret_key');
let decrypted = decipher.update(ciphertext, 'hex', 'utf8');
decrypted += decipher.final('utf8');
return decrypted;
}
const decryptedText = caesarCipherDecrypt(ciphertext, shift);
console.log(decryptedText);
```
请注意,这只是凯撒密码的一个简单示例,实际应用中可能需要更复杂的加密算法和密钥管理方式。
阅读全文
相关推荐
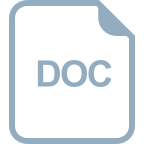
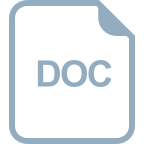
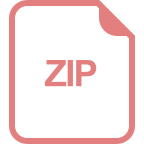
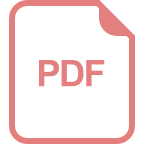
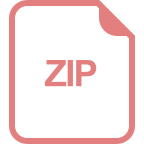
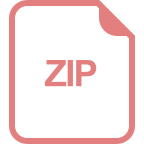
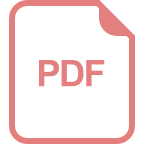
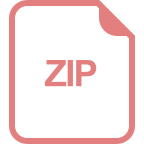
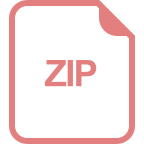
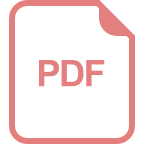
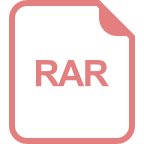
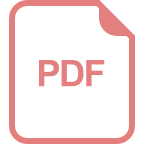
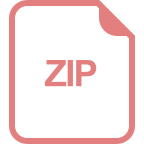
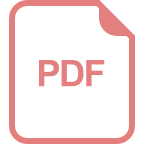
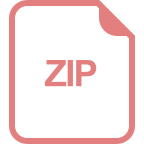
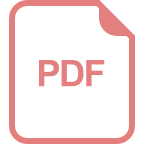
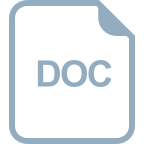
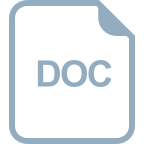