用C#写arraylist任意类型数据排序
时间: 2024-08-14 07:00:37 浏览: 59
在C#中,如果你有一个`ArrayList`存储了不同类型的数据并且需要对其进行排序,你可以使用泛型和`List<T>`替代,因为`ArrayList`并不直接支持泛型并自动排序。以下是使用`List<T>`进行任意类型数据排序的一个例子:
```csharp
using System;
using System.Collections.Generic;
// 定义一个委托类型的比较函数,用于指定排序规则
public delegate bool Comparison<T>(T x, T y);
class Program
{
// 示例:对字符串和整数混合的List进行排序
static void Main()
{
List<object> mixedData = new List<object>()
{
5,
"apple",
2,
"banana",
7,
"orange"
};
// 创建一个实例化后的Comparison委托,这里假设我们按字典序排序字符串,然后是数字升序
Comparison<object> comparison = (x, y) =>
{
if (x is string && y is int)
return ((string)x).CompareTo((string)y);
else if (x is int && y is string)
return ((int)x).CompareTo((int)y);
else if (x is IComparable && y is IComparable)
return (x as IComparable).CompareTo(y as IComparable);
else
throw new ArgumentException("Cannot compare elements of different types");
};
mixedData.Sort(comparison);
Console.WriteLine("Sorted data:");
foreach (var item in mixedData)
{
Console.WriteLine(item);
}
}
}
```
在这个示例中,`comparison`委托用于定义如何比较列表中的元素。注意,为了处理不同类型的数据,你需要提供一些条件判断来确定如何进行比较。
阅读全文
相关推荐
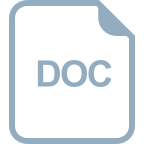
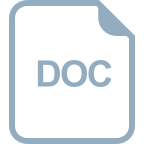
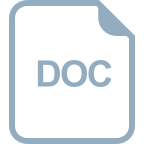

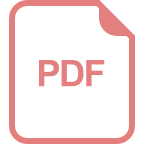
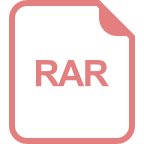
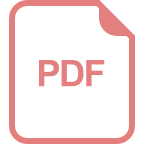
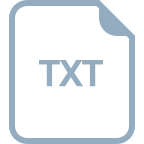
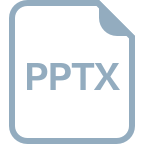
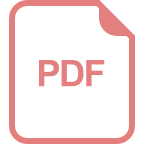
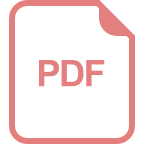
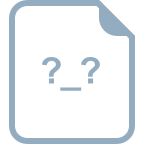
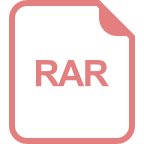
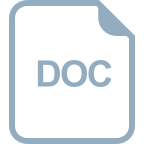
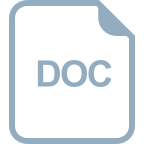
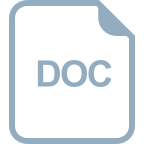


