WPF的DataGrid通过行、列找到确定的单元格
时间: 2024-09-14 16:10:01 浏览: 46
在WPF的DataGrid中,要找到特定的行和列对应单元格,可以使用`DataGridCell`类和其关联的数据项属性。下面是如何操作的一个简单示例[^2]:
```csharp
// 假设你有一个ObservableCollection作为DataGrid的ItemsSource
ICollectionView data = (ICollectionView)dataGrid.ItemsSource;
// 获取指定行和列的单元格
int rowIndex = 0; // 替换为实际行索引
int columnIndex = 1; // 替换为实际列索引
DataGridCell cell = data.ItemAt(rowIndex).FindAncestor<DataGridRow>(true).Children[columnIndex] as DataGridCell;
// 如果找到了单元格,你可以进一步修改它的背景颜色或其他样式属性
if (cell != null)
{
cell.Background = Brushes.Yellow; // 更改背景色为黄色
}
```
这里的关键在于调用`FindAncestor<DataGridRow>(true)`来查找包含指定数据项的行,然后从该行的子元素中获取对应的列。
相关问题
WPF DataGrid根据数据大小判定改变单元格并背景色
如果你想根据数据大小判定来改变单元格的背景色,可以使用 DataTrigger 和 Converter 来实现。以下是一个简单的示例:
1. 在 XAML 中添加 DataGrid 控件,并设置 AutoGenerateColumns 属性为 False。
```xml
<DataGrid x:Name="MyDataGrid" AutoGenerateColumns="False">
<DataGrid.Columns>
<DataGridTextColumn Header="Name" Binding="{Binding Name}" />
<DataGridTextColumn Header="Age" Binding="{Binding Age}" />
<DataGridTextColumn Header="Score" Binding="{Binding Score}" />
</DataGrid.Columns>
</DataGrid>
```
2. 在代码中创建数据源,并设置 DataGrid 的 ItemsSource 属性。
```csharp
List<Person> people = new List<Person>
{
new Person { Name = "Tom", Age = 28, Score = 90 },
new Person { Name = "Jerry", Age = 32, Score = 85 },
new Person { Name = "Mickey", Age = 25, Score = 95 }
};
MyDataGrid.ItemsSource = people;
```
3. 在 DataGrid.CellStyle 属性中定义单元格样式,使用 DataTrigger 和 Converter 来改变单元格背景色。
```xml
<DataGrid.CellStyle>
<Style TargetType="DataGridCell">
<Setter Property="Background" Value="Transparent" />
<Style.Triggers>
<DataTrigger Binding="{Binding Score, Converter={StaticResource ScoreToBrushConverter}}" Value="Red">
<Setter Property="Background" Value="Red" />
</DataTrigger>
<DataTrigger Binding="{Binding Score, Converter={StaticResource ScoreToBrushConverter}}" Value="Yellow">
<Setter Property="Background" Value="Yellow" />
</DataTrigger>
<DataTrigger Binding="{Binding Score, Converter={StaticResource ScoreToBrushConverter}}" Value="Green">
<Setter Property="Background" Value="Green" />
</DataTrigger>
</Style.Triggers>
</Style>
</DataGrid.CellStyle>
```
在上述示例中,我们使用了一个 ScoreToBrushConverter,将 Score 转换为对应的颜色值。Converter 的代码如下:
```csharp
public class ScoreToBrushConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
int score = (int)value;
if (score < 60)
{
return "Red";
}
else if (score < 80)
{
return "Yellow";
}
else
{
return "Green";
}
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
```
在 Converter 中,我们根据 Score 的大小来返回对应的颜色值。在 DataTrigger 中,我们使用了 Converter 来将 Score 转换为颜色值,并根据颜色值来改变单元格的背景色。
需要注意的是,Converter 返回的颜色值可以是 Color、Brush 或字符串类型。在示例中,我们返回的是字符串类型,因为我们使用的是命名颜色,也可以使用 SolidColorBrush 或 LinearGradientBrush 等 Brush 类型来返回。
WPF Datagrid如何通过Storyboard实现单元格闪烁
在WPF中,可以使用Storyboard和DataGrid控件结合,来实现单元格闪烁的效果。首先,你需要创建一个Storyboard来控制动画。以下是基本步骤:
1. **准备XAML**:
```xml
<DataGrid x:Name="myDataGrid">
<!--...数据网格的其他配置...-->
</DataGrid>
<Storyboard x:Key="CellFlashStoryboard">
<DoubleAnimationUsingKeyFrames Duration="0:0:0.5"
Storyboard.TargetProperty="(UIElement.Opacity)">
<EasingDoubleKeyFrame KeyTime="0" Value="1"/>
<EasingDoubleKeyFrame KeyTime="0.5" Value="0"/>
</DoubleAnimationUsingKeyFrames>
</Storyboard>
```
2. **设置触发条件**:
可能需要一个Command或者EventTrigger来启动闪烁。例如,你可以监听`MouseEnter`事件:
```xml
<DataGrid.CellMouseEnter>
<EventTrigger RoutedEvent="DataGrid.CellMouseEnter">
<BeginStoryboard storyboard="{StaticResource CellFlashStoryboard}"/>
</EventTrigger>
</DataGrid.CellMouseEnter>
```
3. **添加透明度动画**:
当鼠标进入单元格时,Storyboard会开始播放,改变该单元格的`Opacity`属性从1逐渐降低到0,达到闪烁效果。
4. **停止动画**:
对于离开单元格的动画,可以在`MouseLeave`事件中添加一个`StopStoryboard`行为,或者在Storyboard内部设置循环次数。
注意:以上示例是一个基础的闪烁动画,实际应用中可能需要调整动画的时间、平滑度等细节。同时,为了节省性能,也可以考虑只对焦点单元格或特定单元格执行闪烁动画。
阅读全文
相关推荐
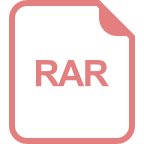
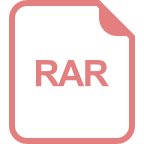
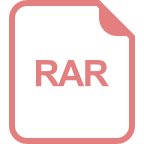











