一个迷宫由R行C列格子组成,有的格子里有障碍物,不能走;有的格子是空地,可以走。 给定一个迷宫,求从左上角走到右下角最少需要走多少步(数据保证一定能走到)。只能在水平方向或垂直方向走,不能斜着走。请根据题目写一段c++代码
时间: 2024-11-03 07:18:27 浏览: 47
这是一个典型的二维空间搜索问题,通常可以用宽度优先搜索(Breadth First Search, BFS)或深度优先搜索(Depth First Search, DFS)解决,这里我们选择BFS因为它的查找顺序更有利于找到最短路径。以下是用C++实现的BFS算法:
```cpp
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
// 定义迷宫的结构体
struct Cell {
int row, col; // 行和列
bool visited; // 是否访问过
};
// 定义邻接矩阵,0表示能走,1表示不能走
const int EMPTY = 0;
const int OBSTACLE = 1;
// 计算从起点到终点的距离
int minSteps(vector<vector<int>>& maze, int startRow, int startCol) {
int rows = maze.size();
int cols = maze[0].size();
vector<vector<bool>> visited(rows, vector<bool>(cols, false));
queue<Cell> q;
q.push({startRow, startCol});
visited[startRow][startCol] = true;
while (!q.empty()) {
Cell current = q.front(); // 取出队首元素
q.pop();
if (current.row == rows - 1 && current.col == cols - 1) { // 到达终点
return current.row + current.col; // 返回步数
}
// 邻居节点
for (int dr = -1; dr <= 1; ++dr) {
for (int dc = -1; dc <= 1; ++dc) {
int newRow = current.row + dr;
int newCol = current.col + dc;
// 如果越界、障碍或已访问,则跳过
if (newRow < 0 || newRow >= rows || newCol < 0 || newCol >= cols ||
maze[newRow][newCol] == OBSTACLE || visited[newRow][newCol]) {
continue;
}
visited[newRow][newCol] = true;
q.push({newRow, newCol}); // 将邻居加入队列
}
}
}
return -1; // 如果未到达终点,返回-1表示无法到达
}
int main() {
// 测试示例
vector<vector<int>> maze = {{0, 0, 1}, {0, 1, 0}, {1, 0, 0}}; // 3x3迷宫,障碍物用1表示
int startRow = 0, startCol = 0; // 起点
cout << "Minimum steps to reach the bottom right corner: " << minSteps(maze, startRow, startCol) << endl;
return 0;
}
```
阅读全文
相关推荐









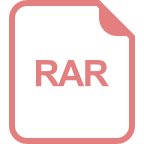
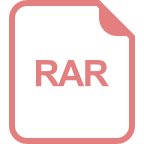







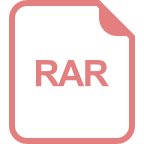