python chain
时间: 2024-10-01 21:01:24 浏览: 45
`chain()` 是 Python 中 `itertools` 模块中的一个函数,它主要用于连接两个或更多可迭代的对象(如列表、元组或字符串),形成一个新的迭代器,返回的是每个可迭代对象的第一个元素,直到所有输入序列都被遍历完毕[^1]。与直接合并这些列表不同,`chain()` 不会创建新的列表或增加内存负担。
下面是一些关于 `chain()` 的使用示例:
1. 对单个可迭代对象进行迭代:
```python
from itertools import chain
li = ['ABC', 'DEF', 'GHI', 'JKL']
res1 = list(chain(li)) # 直接使用 chain
# 结果: ['ABC', 'DEF', 'GHI', 'JKL']
res2 = list(chain.from_iterable(li)) # 使用 chain.from_iterable
# 结果: ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L']
```
2. 连接多个可迭代对象[^2]:
```python
a = [1, 2, 3, 4]
b = ['x', 'y', 'z']
for x in chain(a, b):
print(x)
# 输出: 1, 2, 3, 4, x, y, z
```
通过这种方式,你可以轻松地在单次循环中处理来自多个源的数据。
相关问题
python的chain
Python的`itertools`模块中提供了`chain`函数,它可以将多个可迭代对象合并为一个更大的可迭代对象,按顺序排列。
使用`chain`函数可以避免在多个可迭代对象之间频繁地进行转换和拼接操作。下面是使用示例:
```python
from itertools import chain
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
merged_list = list(chain(list1, list2, list3))
print(merged_list)
# 输出 [1, 2, 3, 4, 5, 6, 7, 8, 9]
```
在上述示例中,`chain`函数将三个列表合并为一个更大的列表,并将其赋值给`merged_list`变量。这个变量可以像普通列表一样进行操作。
Python中chain函数
Python中的`itertools.chain()`函数可以将多个可迭代对象连接起来,形成一个更大的可迭代对象。这个函数接收任意数量的可迭代对象,然后返回一个迭代器。
例如,我们可以使用`chain()`函数将两个列表连接起来:
```
from itertools import chain
list1 = [1, 2, 3]
list2 = [4, 5, 6]
combined_list = list(chain(list1, list2))
print(combined_list) # 输出 [1, 2, 3, 4, 5, 6]
```
`chain()`函数会将`list1`和`list2`中的元素连接起来,形成一个新的可迭代对象。在上面的例子中,我们使用了`list()`函数将迭代器转换为了一个列表。
`chain()`函数可以接收任意数量的可迭代对象,不仅仅是两个列表。它们可以是列表、元组、集合、字符串,或者是生成器等任何可迭代对象。
阅读全文
相关推荐
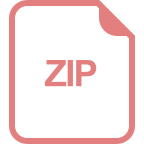
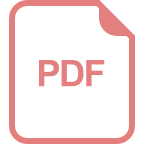
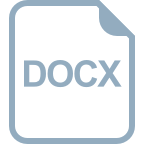
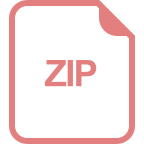
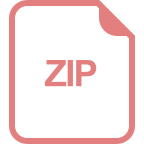
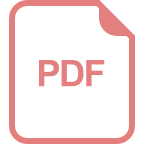
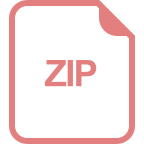
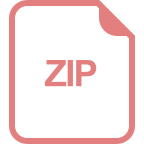
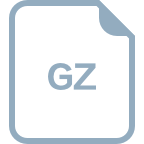
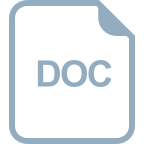
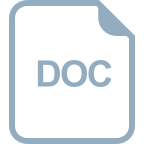
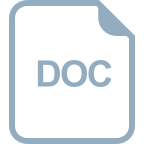
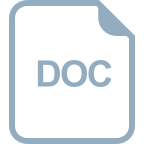
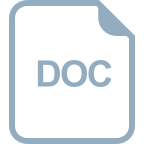
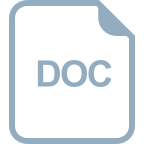