python机考常用函数
时间: 2025-01-07 13:06:48 浏览: 5
### Python 机考常用函数
#### 内置函数概述
Python 提供了大量的内置函数,在编程考试中,掌握这些函数能够显著提高编码效率。官方文档列出了69个内置函数,其中一些是在日常开发中最常使用的[^1]。
#### 频繁使用的八个内置函数及其应用实例
##### `len()` 获取长度
`len()` 函数用于获取字符串、列表、元组等序列类型的长度。
```python
example_list = ['apple', 'banana', 'cherry']
print(len(example_list)) # 输出:3
```
##### `type()` 和 `isinstance()` 类型判断
这两个函数可以帮助程序员确认变量的数据类型。
```python
variable = "hello"
print(type(variable)) # <class 'str'>
print(isinstance(variable, str)) # True
```
##### `sum()`, `max()`, `min()` 数学运算
这三个函数分别用于求和、寻找最大值和最小值。
```python
numbers = [1, 2, 3, 4, 5]
print(sum(numbers)) # 输出:15
print(max(numbers)) # 输出:5
print(min(numbers)) # 输出:1
```
##### `sorted()` 排序操作
此函数不仅可以对简单数据结构进行排序,还可以通过指定参数实现更复杂的排序逻辑,比如与 lambda 表达式结合使用来定制化排序规则[^3]。
```python
data_points = [(1,'b'),(3,'a'),(2,'c')]
sorted_data = sorted(data_points, key=lambda x: (x[0], x[1]))
print(sorted_data) # 输出:[(1, 'b'), (2, 'c'), (3, 'a')]
```
##### `map()`, `filter()`, `reduce()` 数据处理工具
这是一系列高阶函数,适用于集合上的批量转换或筛选工作;注意 `reduce()` 需要从 functools 导入。
```python
from functools import reduce
items = [1, 2, 3, 4]
squared_items = list(map(lambda x: x ** 2, items))
even_items = list(filter(lambda x: not x % 2, squared_items))
product_of_evens = reduce((lambda x, y: x * y), even_items)
print(squared_items) # 输出:[1, 4, 9, 16]
print(even_items) # 输出:[4, 16]
print(product_of_evens) # 输出:64
```
##### `Counter()` 统计频率
来自 collections 库的 Counter 工具可以方便地计算出给定容器内各元素的数量分布情况[^2]。
```python
from collections import Counter
nums = [1, 1, 1, 6, 6, 6, 7, 8]
count = Counter(nums)
for k, v in count.items():
print(f"{k}: {v}")
# 输出:
# 1: 3
# 6: 3
# 7: 1
# 8: 1
```
阅读全文
相关推荐










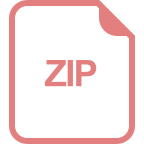








