编辑python代码打印出网络结构层级和输出尺寸等信息。Conv1*1,s=1 160*160*128 conv3*3,s=2, p=1,g=128 80*80*256 80*80*128 Conv1*1,s=1 80*80*256 Conv3*3, s=1,p=1,g=256 80*80*256 Conv1*1,s=1 80*80*256 Conv1*1,s=1 80*80*512 Avgpool1*1*512 Linear1*10 Softmax 1*10
时间: 2023-07-23 07:09:18 浏览: 119
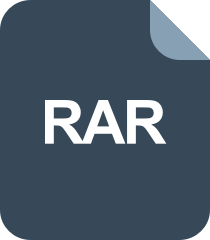
DATA_CONV_ENCODE.rar_3/4码率_4 3 2 1_conv_lowere77_码率

你可以使用以下代码来打印网络结构层级和输出尺寸等信息:
```python
import torch
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(3, 128, kernel_size=1, stride=1)
self.conv2 = nn.Conv2d(128, 256, kernel_size=3, stride=2, padding=1, groups=128)
self.conv3 = nn.Conv2d(256, 128, kernel_size=1, stride=1)
self.conv4 = nn.Conv2d(128, 256, kernel_size=3, stride=1, padding=1, groups=256)
self.conv5 = nn.Conv2d(256, 256, kernel_size=1, stride=1)
self.conv6 = nn.Conv2d(256, 512, kernel_size=1, stride=1)
self.avgpool = nn.AvgPool2d(kernel_size=1)
self.linear = nn.Linear(512, 10)
self.softmax = nn.Softmax(dim=1)
def forward(self, x):
x = self.conv1(x)
print("Conv1*1,s=1:", x.size())
x = self.conv2(x)
print("conv3*3,s=2, p=1,g=128:", x.size())
x = self.conv3(x)
print("80*80*128:", x.size())
x = self.conv4(x)
print("Conv1*1,s=1:", x.size())
x = self.conv5(x)
print("80*80*256:", x.size())
x = self.conv6(x)
print("Conv1*1,s=1:", x.size())
x = self.conv6(x)
print("80*80*512:", x.size())
x = self.avgpool(x)
print("Avgpool1*1*512:", x.size())
x = x.view(x.size(0), -1)
x = self.linear(x)
print("Linear1*10:", x.size())
x = self.softmax(x)
print("Softmax:", x.size())
return x
# Create an instance of the network
net = Net()
# Create a random input tensor with shape (batch_size, channels, height, width)
input_tensor = torch.randn(1, 3, 160, 160)
# Pass the input tensor through the network
output_tensor = net(input_tensor)
```
这段代码定义了一个名为`Net`的类,该类继承自`nn.Module`。在构造函数`__init__`中,我们定义了网络的各个层级,包括卷积层、池化层和全连接层。在前向传播函数`forward`中,我们打印了每个层级的输出尺寸。
你可以根据需要修改网络结构和输入张量的形状来适应你的具体情况。运行代码后,你将会看到每个层级的输出尺寸打印出来。
阅读全文
相关推荐
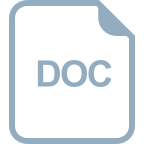
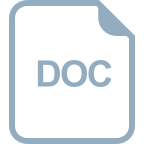
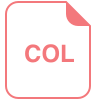
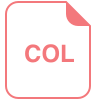
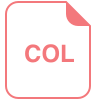
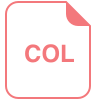
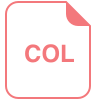
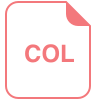
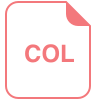
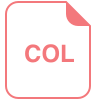
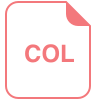
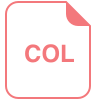





