C语言 函数 形参 指针
时间: 2024-11-08 22:12:20 浏览: 44
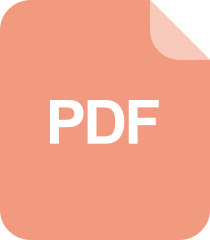
深入解析C语言中函数指针的定义与使用
在C语言中,函数是组织代码块的一种结构,可以接收输入并返回结果。形参(Parameters)是函数定义中的变量,它们用于接收函数调用时传递的值。当我们在函数名后面加上圆括号并提供一些数据(即实际参数),这些数据实际上是通过指针(Pointers)间接传递给形参的。
C函数中的形参有两种常见类型:直接传递和通过指针传递。1. 直接传递:如果形参类型与实际参数类型一致,C会自动复制数据,不需要显式使用指针。例如:
```c
void print(int x) {
printf("%d", x);
}
print(5); // 直接将整数5传给x
```
2. 通过指针传递:当我们想修改函数内部的形参时,通常使用指针。通过将指针地址作为实参,可以在函数内操作指向的数据。例如:
```c
void increment(int *ptr) {
(*ptr)++;
}
int main() {
int num = 5;
increment(&num); // 传递num的地址,函数内部会增加num的值
printf("%d", num); // 输出6
}
```
通过指针,我们可以实现更灵活的数据共享,并控制对原始数据的操作。
阅读全文
相关推荐
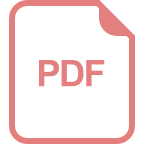
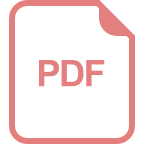

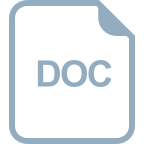
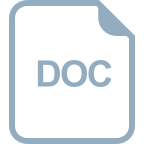




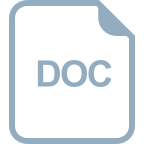
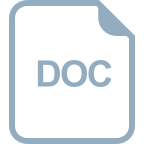
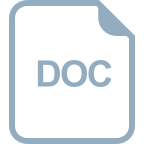





