Python+pgsql
时间: 2023-12-27 16:04:47 浏览: 40
Python是一种流行的编程语言,而pgsql是一种流行的关系型数据库管理系统。Python和pgsql可以很好地结合使用,以便在Python应用程序中使用数据库。以下是Python和pgsql结合使用的一些方法:
1.使用psycopg2库连接到pgsql数据库并执行查询:
```python
import psycopg2
# 连接到数据库
conn = psycopg2.connect(database="mydatabase", user="myusername", password="mypassword", host="localhost", port="5432")
# 执行查询
cur = conn.cursor()
cur.execute("SELECT * FROM mytable")
rows = cur.fetchall()
# 处理结果
for row in rows:
print(row)
# 关闭连接
conn.close()
```
2.使用SQLAlchemy库连接到pgsql数据库并执行查询:
```python
from sqlalchemy import create_engine
# 连接到数据库
engine = create_engine('postgresql://myusername:mypassword@localhost:5432/mydatabase')
# 执行查询
result = engine.execute('SELECT * FROM mytable')
# 处理结果
for row in result:
print(row)
# 关闭连接
engine.dispose()
```
3.使用Django ORM连接到pgsql数据库并执行查询:
```python
from django.db import connection
# 执行查询
with connection.cursor() as cursor:
cursor.execute("SELECT * FROM mytable")
rows = cursor.fetchall()
# 处理结果
for row in rows:
print(row)
```
相关推荐
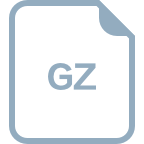














