y=3x+2,-x+1 x大于等于0 求输出y的值
时间: 2024-10-26 11:04:02 浏览: 35
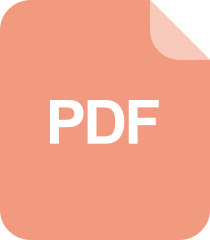
在python中实现求输出1-3+5-7+9-……101的和

在C语言中,如果你想根据条件来计算`y`的值(当`x >= 0`时,`y = -x + 1`),你可以使用`if`语句来实现。以下是如何编写这个功能的简单示例:
```c
#include <stdio.h>
double calculate_y(double x) {
if (x >= 0) {
return -x + 1;
} else {
// 这里不需要,因为我们已经处理了x>=0的情况
// 如果x<0,y会默认按照3x+2计算,因为代码中未做特别规定
return 3 * x + 2;
}
}
int main() {
double input_x;
printf("Enter a value for x: ");
scanf("%lf", &input_x);
double output_y = calculate_y(input_x);
printf("For x=%lf, the calculated y value is: %lf\n", input_x, output_y);
return 0;
}
```
当你运行这段程序并输入一个非负数时,它会输出`y`的值;如果输入的是负数,那么它会按`3x + 2`的公式计算。
阅读全文
相关推荐
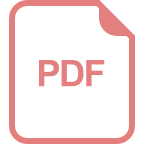
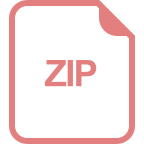
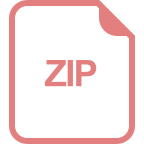
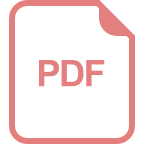
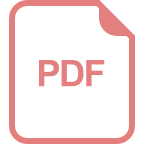
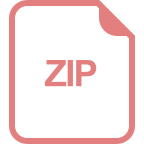
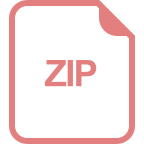
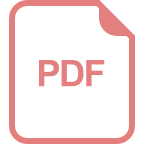
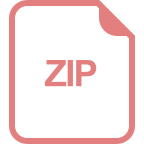
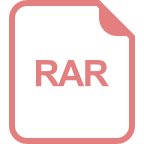
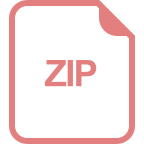
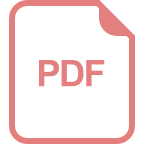
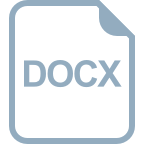
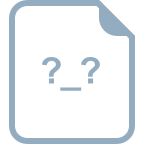
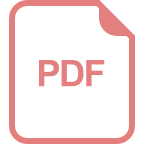
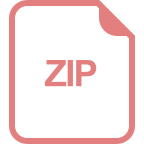
