用JAVE语言输出一组公司员工的信息列表,其中员工具备以下特征: 雇员:员工编号,姓名,工资。 经理:员工编号,姓名,工资,管理部门 总监:员工编号,姓名,工资,津贴
时间: 2024-11-24 08:42:01 浏览: 8
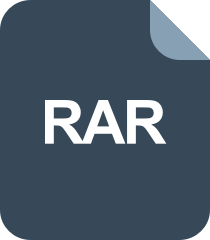
JAVE-SMS.rar_java 短信猫_jave_jindisms-3.1-RC1.jar_sms_短信猫
在Java中,你可以使用类和对象的概念来创建一个员工信息列表,每个员工可以是雇员、经理或总监,并包含相应的属性。首先,我们定义一个通用的`Employee`类作为基础:
```java
public class Employee {
private int employeeId;
private String name;
private double salary;
// 构造函数
public Employee(int id, String name, double salary) {
this.employeeId = id;
this.name = name;
this.salary = salary;
}
// getter和setter方法
public int getEmployeeId() { return employeeId; }
public void setEmployeeId(int employeeId) { this.employeeId = employeeId; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public double getSalary() { return salary; }
public void setSalary(double salary) { this.salary = salary; }
}
```
然后,我们可以分别为经理和总监添加额外的属性和一个管理部属性:
```java
public class Manager extends Employee {
private String department;
// 构造函数
public Manager(int id, String name, double salary, String department) {
super(id, name, salary);
this.department = department;
}
public String getDepartment() { return department; }
public void setDepartment(String department) { this.department = department; }
}
public class Director extends Employee {
private double bonus;
// 构造函数
public Director(int id, String name, double salary, double bonus) {
super(id, name, salary);
this.bonus = bonus;
}
public double getBonus() { return bonus; }
public void setBonus(double bonus) { this.bonus = bonus; }
}
```
现在,你可以创建一个`List<Employee>`来存储这些对象,并根据需要打印员工信息:
```java
public static void main(String[] args) {
List<Employee> employees = new ArrayList<>();
employees.add(new Manager(1, "张三", 8000, "销售部"));
employees.add(new Director(2, "李四", 15000, 5000, "市场部"));
for (Employee employee : employees) {
System.out.println("员工ID: " + employee.getEmployeeId());
System.out.println("姓名: " + employee.getName());
System.out.println("工资: " + employee.getSalary());
if (employee instanceof Manager) {
Manager manager = (Manager) employee;
System.out.println("部门: " + manager.getDepartment());
} else if (employee instanceof Director) {
Director director = (Director) employee;
System.out.println("津贴: " + director.getBonus());
}
System.out.println();
}
}
```
阅读全文
相关推荐
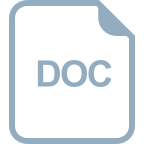
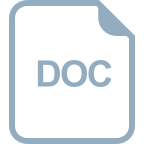

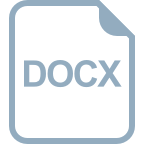
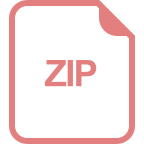
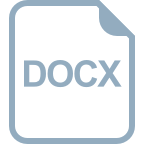
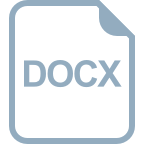
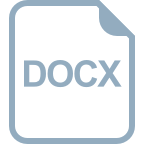
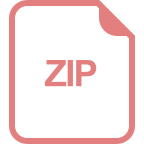




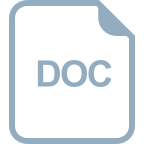
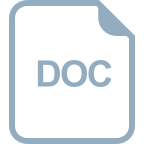
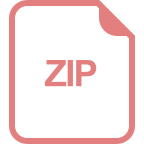