python函数与抽象编程实践——交通信号灯模拟:实现以下功能:1. 交通信号灯(红绿灯)的切换。2.人行道信号灯(红绿灯)的切换。3.实时显示当前信号灯状态和倒计时。4.对程序进行优化,增加黄灯状态的相关逻辑。
时间: 2024-12-25 11:34:09 浏览: 13
Python 函数可以很好地应用于抽象编程实践中,比如模拟交通信号灯系统。下面是一个简单的模拟例子,使用类定义红绿灯和人行道信号灯,以及相关的状态转换、倒计时和显示:
```python
import time
class TrafficLight:
def __init__(self):
self.colors = ['red', 'yellow', 'green']
self.current_state = self.colors[0] # 初始状态为红色
self.timer = 60 # 每个颜色持续时间设为60秒
def switch(self):
if self.current_state == 'red':
self.current_state = 'green'
elif self.current_state == 'green':
self.current_state = 'yellow'
else: # yellow state
self.current_state = 'red'
def countdown(self):
while self.timer > 0:
print(f"当前信号灯状态:{self.current_state},剩余时间:{self.timer}秒")
time.sleep(1)
self.timer -= 1
self.switch()
class PedestrianLight(TrafficLight):
# 子类,用于处理人行道信号灯
def __init__(self):
super().__init__()
self.pedestrian_timer = 15 # 人行横道绿灯时间为15秒
def pedestrian_crossing(self):
if self.current_state == 'green' and self.is_pedestrian():
print("行人可以过马路了,绿灯时间:", self.pedestrian_timer)
self.countdown()
else:
print("行人等待")
def is_pedestrian(self): # 这里可以根据实际需求设计判断逻辑
return self.current_state == 'green'
if __name__ == "__main__":
traffic_light = TrafficLight()
traffic_light.countdown()
pedestrian_light = PedestrianLight()
pedestrian_light.pedestrian_crossing()
```
在这个例子中,我们创建了两个类 `TrafficLight` 和 `PedestrianLight`,分别代表常规的交通信号灯和人行道信号灯。`switch` 方法实现了红绿灯的切换,`countdown` 和 `pedestrian_crossing` 分别负责正常倒计时和行人通行逻辑。通过子类 `PedestrianLight`,我们可以扩展特定的功能。
阅读全文
相关推荐
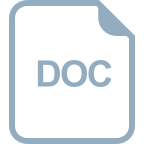
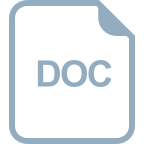
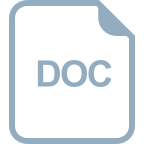
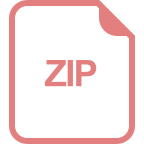
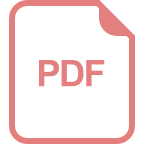
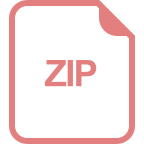
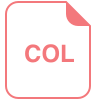
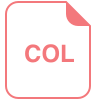
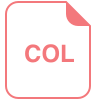
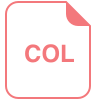
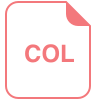
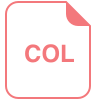
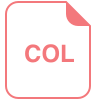
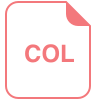
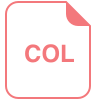
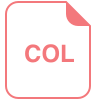
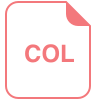
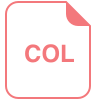
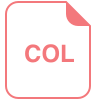