用Python实现一个16.图像处理工具 要求:对图像进行基本处理,如裁剪、缩放、旋转、添加滤镜等。或在图像上添加透明水印,以保护版权或品牌标识。
时间: 2024-10-16 07:04:32 浏览: 50
在Python中,可以使用PIL(Pillow)库来进行图像处理,这是一个功能强大的库,常用于各种基础操作以及更复杂的图像分析任务。以下是使用PIL库实现一些基本图像处理步骤:
1. 安装Pillow:
```bash
pip install Pillow
```
2. **裁剪**:
```python
from PIL import Image
img = Image.open('image.jpg')
cropped_img = img.crop((left, top, right, bottom))
cropped_img.save('cropped_image.jpg')
```
其中 `(left, top)` 是裁剪区域的左上角坐标,`(right, bottom)` 是右下角坐标。
3. **缩放**:
```python
resized_img = img.resize((new_width, new_height), Image.ANTIALIAS)
resized_img.save('resized_image.jpg')
```
4. **旋转**:
```python
rotated_img = img.rotate(angle, expand=True)
rotated_img.save('rotated_image.jpg')
```
`angle` 是旋转角度,`expand=True` 保持原始尺寸并填充背景色。
5. **添加滤镜**(如模糊、锐化):
```python
blurred_img = img.filter(ImageFilter.BLUR) # 模糊效果
sharpened_img = img.filter(ImageFilter.SHARPEN) # 锐化效果
```
6. **添加透明水印**(文字或图片):
```python
from PIL import ImageDraw, ImageFont
watermark_text = "Copyright"
draw = ImageDraw.Draw(img)
font = ImageFont.truetype('arial.ttf', size=30)
draw.text((10, 10), watermark_text, fill=(255, 255, 255), font=font)
watermark_img_path = 'watermarked_image.jpg'
img.save(watermark_img_path)
```
以上就是使用PIL库在Python中进行基础图像处理的一些示例。记得在实际应用中替换相应的文件路径和参数。
阅读全文
相关推荐





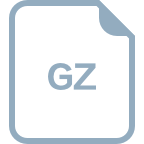
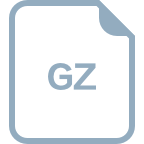










