c#将List序列化保存
时间: 2024-12-26 09:30:04 浏览: 5
在 C# 中,你可以使用 `System.IO` 命名空间下的 `Serializer` 或者 `JsonSerializer` 来序列化(转换成文本格式)和反序列化(从文本格式恢复)一个 `List<T>` 对象。以下是使用 JSON 格式(`.NET DataContract Json Serializer`)序列化的示例:
```csharp
using System;
using System.Collections.Generic;
using System.IO;
using System.Runtime.Serialization.Json;
class Program
{
public class Person
{
public string Name { get; set; }
}
static void Main(string[] args)
{
List<Person> people = new List<Person>
{
new Person { Name = "Alice" },
new Person { Name = "Bob" },
};
// 序列化到文件
string jsonString = JsonConvert.SerializeObject(people);
using (StreamWriter writer = new StreamWriter("people.json"))
{
writer.Write(jsonString);
}
Console.WriteLine("Serialized to file.");
}
}
```
在这个例子中,我们首先将 `List<Person>` 转换为 JSON 字符串,然后写入到名为 "people.json" 的文件中。
如果你想要读取之前保存的数据,可以这样做:
```csharp
// 反序列化从文件加载的数据
string jsonData = File.ReadAllText("people.json");
List<Person> deserializedPeople = JsonConvert.DeserializeObject<List<Person>>(jsonData);
foreach (Person person in deserializedPeople)
{
Console.WriteLine(person.Name);
}
```
阅读全文
相关推荐
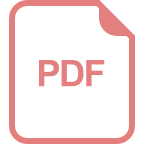
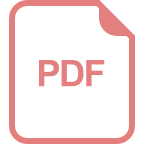
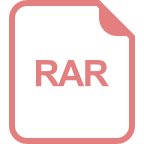
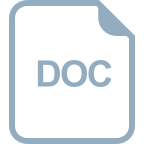
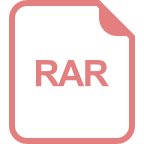
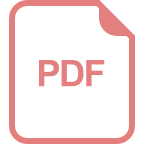
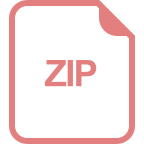
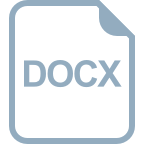
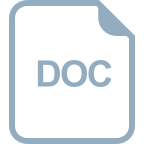
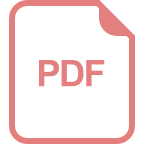
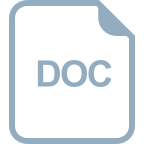
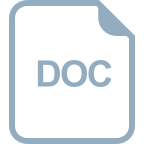
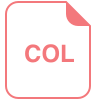





