请用【java】帮我写一段汽车销售统计
时间: 2024-10-15 12:12:58 浏览: 10
在Java中,我们可以创建一个简单的汽车销售统计类来模拟这个场景。首先,我们可以定义一个`Car`接口,然后创建几个实现了这个接口的具体汽车类,比如`Sedan`, `SUV`, 和 `ElectricCar`。接着,我们有一个`SalesReport`类来跟踪销售数据。
```java
// 定义Car接口
public interface Car {
String getCarType();
int getPrice();
}
// 具体汽车类实现
public class Sedan implements Car {
private String type;
private int price;
// 构造函数、getter和setter省略...
public String getCarType() { return type; }
public int getPrice() { return price; }
}
public class SUV extends Sedan {
// SUV类可能额外有一些特性,如越野能力
// ...
}
public class ElectricCar implements Car {
private String type;
private int price;
// 构造函数、getter和setter省略...
// 电动车型可能会有特殊的电池续航信息
// ...
}
// 销售报告类
public class SalesReport {
private Map<String, Integer> salesData = new HashMap<>();
public void sellCar(Car car) {
salesData.put(car.getCarType(), salesData.getOrDefault(car.getCarType(), 0) + car.getPrice());
}
public int getTotalSales(String carType) {
return salesData.getOrDefault(carType, 0);
}
public int getTotalSales() {
int total = 0;
for (int sale : salesData.values()) {
total += sale;
}
return total;
}
}
// 使用示例
public static void main(String[] args) {
SalesReport report = new SalesReport();
report.sellCar(new Sedan()); // 卖出一辆轿车
report.sellCar(new SUV()); // 卖出一辆SUV
report.sellCar(new ElectricCar()); // 卖出一辆电动车
System.out.println("Sedan总销量: " + report.getTotalSales("Sedan"));
System.out.println("所有车型总销量: " + report.getTotalSales());
}
```
阅读全文
相关推荐
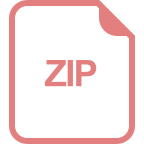
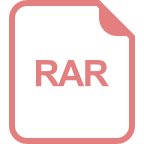
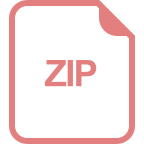
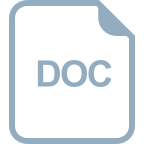
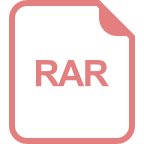
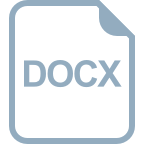
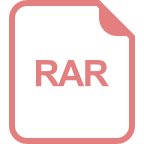
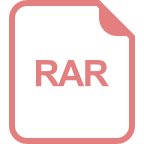
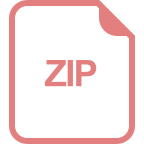
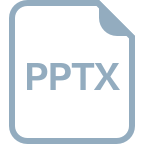
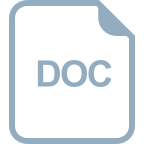
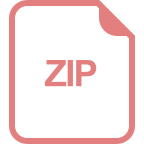
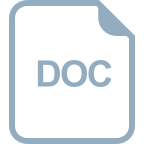
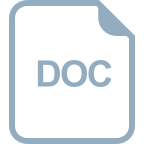
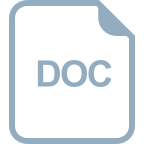
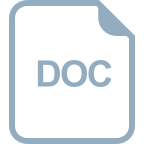
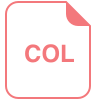
