.请编写一个爬虫,爬取任意一个公开的网页数据,并将爬取到的数据存储为TXT文本文件。
时间: 2024-12-01 16:30:40 浏览: 20
编写一个简单的网页爬虫可以分为以下几个步骤,这里以Python的requests和BeautifulSoup库为例:
1. **安装必要的库**:
首先,确保已经安装了`requests`和`beautifulsoup4`库,如果没有安装,可以通过pip进行安装:
```
pip install requests beautifulsoup4
```
2. **获取网页内容**:
使用`requests.get()`函数发送HTTP请求并获取页面的HTML内容:
```python
import requests
url = "https://example.com" # 替换为你想要爬取的网站地址
response = requests.get(url)
html_content = response.text
```
3. **解析网页内容**:
使用BeautifulSoup解析HTML文档,提取需要的信息:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
data_to_save = soup.find_all('tag_name') # 将'tag_name'替换为目标标签名
```
4. **保存数据到TXT文件**:
将提取的数据写入TXT文件:
```python
with open('output.txt', 'w', encoding='utf-8') as f:
for item in data_to_save:
f.write(str(item) + '\n')
```
5. **异常处理**:
添加适当的错误处理,比如网络连接失败、请求超时等情况。
注意,爬取网页时要遵守网站的robots.txt规则,并尊重网站所有权,不要对目标网站造成过大负担。
阅读全文
相关推荐
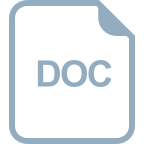
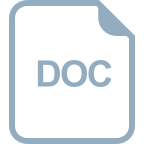
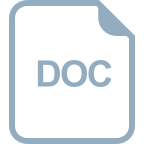
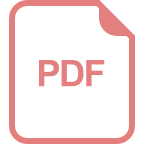
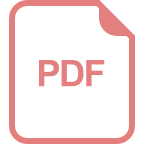
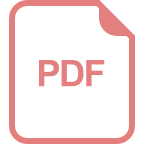
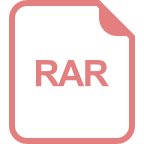
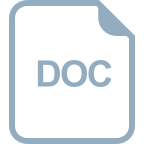
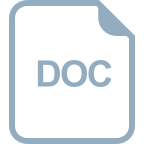
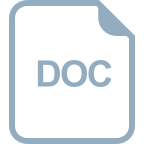
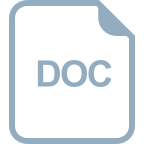
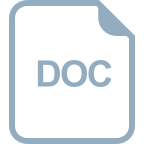
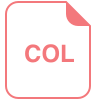
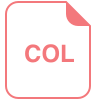
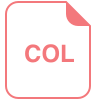
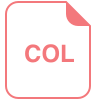
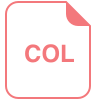
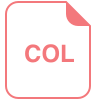
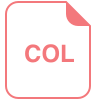