java数据结构栈的链式存储及基本算数运算算法实现
时间: 2024-11-22 13:39:25 浏览: 11
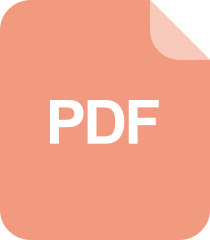
Java栈之链式栈存储结构的实现代码
在Java中,栈是一种线性数据结构,通常采用链表的方式来实现。链式存储的栈,也叫做单链栈,它由两部分组成:栈顶节点(Top Node)和一个指向栈顶节点的指针。当元素入栈(push)时,新元素会被添加到栈顶,并更新顶部指针;出栈(pop)操作则会移除并返回栈顶元素,同时更新顶部指针。
下面是一个简单的链式栈的基本算术运算算法实现(这里假设我们只做加法运算):
```java
class StackNode {
int value;
StackNode next;
// 构造函数和默认值省略
}
public class LinkedStack {
private StackNode top; // 栈顶节点
// 入栈操作
public void push(int num) {
StackNode newNode = new StackNode();
newNode.value = num;
newNode.next = top;
top = newNode;
}
// 出栈操作(如果为空抛出异常)
public int pop() throws EmptyStackException {
if (top == null) {
throw new EmptyStackException();
}
int result = top.value;
top = top.next;
return result;
}
// 检查栈是否为空
public boolean isEmpty() {
return top == null;
}
// 遇到算术运算时,可以在这里添加一个计算函数
public int calculateSum(int num1, int num2) throws EmptyStackException {
if (isEmpty()) {
throw new EmptyStackException();
}
StackNode temp = top;
top = top.next;
int sum = temp.value + num2;
temp.value = sum; // 更新栈顶节点,模拟将num2入栈
return num1 + sum; // 返回最终结果,相当于num1 + (num2 + top.value)
}
}
```
阅读全文
相关推荐
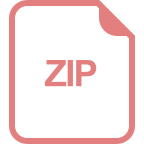
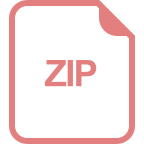
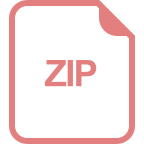
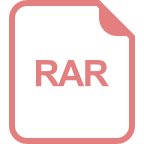
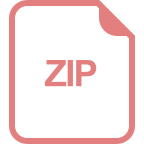
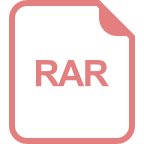
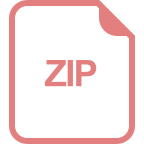
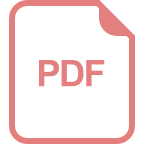
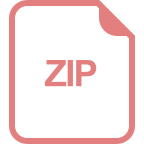
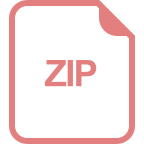
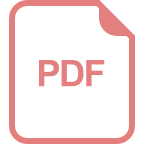
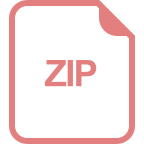
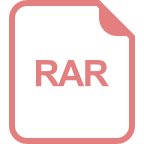
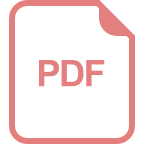