python列表案例
时间: 2024-09-13 19:01:14 浏览: 41
Python列表是一种可变的序列类型,可以用来存储多个元素,其中的元素可以是不同类型的数据。列表是Python中最常用的数据结构之一,以下是一些使用Python列表的基本案例:
1. 创建列表:
```python
fruits = ['apple', 'banana', 'cherry']
numbers = [1, 2, 3, 4, 5]
empty_list = []
```
2. 访问列表元素:
```python
print(fruits[0]) # 输出 'apple'
print(numbers[-1]) # 输出 5
```
3. 列表切片:
```python
print(fruits[1:3]) # 输出 ['banana', 'cherry']
```
4. 列表元素的增加与删除:
```python
fruits.append('orange') # 在列表末尾添加一个元素
fruits.insert(1, 'strawberry') # 在索引1的位置插入 'strawberry'
fruits.pop(2) # 移除索引2的元素
fruits.remove('apple') # 移除列表中的 'apple'
```
5. 列表排序:
```python
numbers.sort() # 升序排序
numbers.sort(reverse=True) # 降序排序
```
6. 列表推导式:
```python
squares = [x**2 for x in numbers]
```
7. 多维列表:
```python
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
```
8. 列表与其他序列类型的转换:
```python
tuple_to_list = list((1, 2, 3))
list_to_string = ''.join(fruits)
```
阅读全文
相关推荐
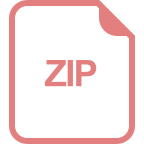
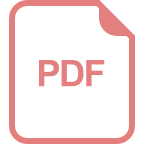
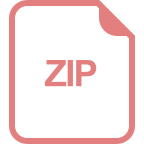
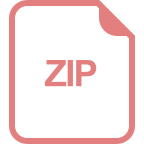
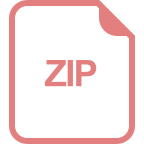
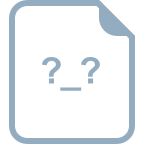
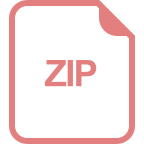
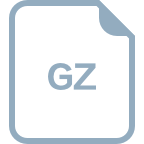
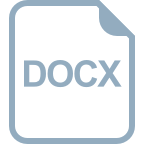
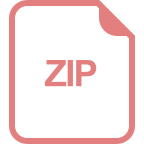
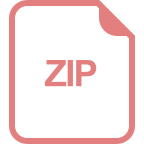
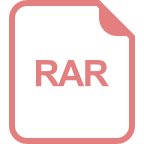
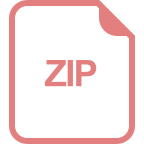
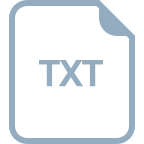