用数组实现栈的进栈(push),出栈(pop)和栈中元素的输出(display)
时间: 2024-10-16 12:21:23 浏览: 5
在C++中,使用数组实现栈(Stack)的基本操作包括进栈(push)、出栈(pop)以及查看栈顶元素(display)。这里是一个简单的例子,我们将使用固定大小的数组来实现这个栈:
```cpp
#include <iostream>
const int MAX_SIZE = 50; // 栈的最大容量
class Stack {
private:
int items[MAX_SIZE]; // 数组用于存储栈中的元素
int top; // 指针,表示栈顶元素的位置
public:
// 初始化栈为空
Stack() : top(-1) {}
// 判断栈是否已满
bool is_full() {
return (top == MAX_SIZE - 1);
}
// 判断栈是否为空
bool is_empty() {
return (top == -1);
}
// 进栈操作(push)
void push(int value) {
if (!is_full()) {
items[++top] = value;
std::cout << "Pushed element " << value << std::endl;
} else {
std::cout << "Stack is full, cannot push." << std::endl;
}
}
// 出栈操作(pop)
void pop() {
if (!is_empty()) {
top--;
std::cout << "Popped element: " << items[top + 1] << std::endl; // 假设top初始化为-1,实际访问时加1
} else {
std::cout << "Stack is empty, nothing to pop." << std::endl;
}
}
// 显示栈顶元素(display)
int display() {
if (!is_empty()) {
return items[top + 1]; // 注意栈顶索引加1
} else {
return -1; // 表示栈空
}
}
};
int main() {
Stack s;
s.push(1);
s.push(2);
s.push(3);
s.display(); // 输出3
s.pop();
s.display(); // 输出2
return 0;
}
```
在这个例子中,我们创建了一个`Stack`类,其中包含私有成员变量`items`和`top`。`push`方法检查栈是否满了,如果不满就将元素添加到栈顶;`pop`方法检查栈是否为空,如果不空则弹出栈顶元素;`display`方法返回栈顶元素,但不会真的移除它。
需要注意的是,这个实现没有处理栈溢出或下标越界的情况,实际应用中可能需要添加额外的错误检查。此外,为了方便起见,这里假设`top`初始值为-1,但在访问数组元素时应加上1。如果你需要动态扩展栈,那么还需要考虑其他数据结构如链表。
相关推荐
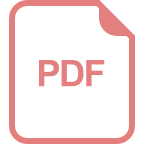
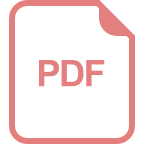
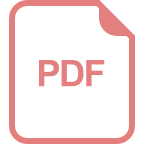















