正方形(square)具有菱形(diamond)的四条边相等的特征,同时又具有长方形(rectangle)四个角是直角的特征。请编写程序实现上述图形的继承关系,输出正方形的特征:四个角是直角、四条边相等。
时间: 2024-09-13 22:02:52 浏览: 12
在Java中,我们可以使用继承机制来创建这种图形的关系。假设我们有一个名为`Shape`的基类,表示所有形状的基本属性和行为,然后派生出两个子类`Rectangle`(长方形)和`Square`(正方形)。因为正方形既是菱形又是长方形的一种特殊情况,所以我们可以在`Square`类中继承自`Rectangle`并且添加额外的菱形特征。
首先,我们定义一个抽象基类`Shape`:
```java
public abstract class Shape {
// 基本属性,如位置(x, y)
protected int x;
protected int y;
// 抽象方法,表示有四个角
public abstract boolean hasRightAngles();
// 具体行为,如计算面积
protected abstract double calculateArea();
}
```
接着,创建`Rectangle`类:
```java
public class Rectangle extends Shape {
private int width;
private int height;
public Rectangle(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
@Override
public boolean hasRightAngles() {
return true; // 长方形的每个角都是90度
}
@Override
protected double calculateArea() {
return width * height;
}
}
```
最后,创建`Square`类:
```java
public class Square extends Rectangle {
// 正方形的边长相等
private int sideLength;
public Square(int x, int y, int sideLength) {
super(x, y, sideLength, sideLength);
this.sideLength = sideLength;
}
@Override
public boolean hasRightAngles() {
return super.hasRightAngles(); // 从父类继承右角直角的特性
}
// 如果需要,这里可以重写calculateArea()来简化计算
@Override
protected double calculateArea() {
return sideLength * sideLength;
}
}
```
现在你可以创建并测试`Square`对象来输出其特性:
```java
public static void main(String[] args) {
Square square = new Square(0, 0, 5);
System.out.println("Square has right angles: " + square.hasRightAngles());
System.out.println("Square's sides are equal: " + square.calculateArea() + " units^2");
}
```
相关推荐
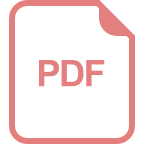
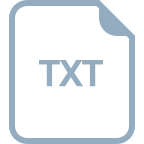
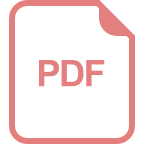














