已知线性表L(x1, x2, ......, xn)各元素递增有序排列,采用顺序存储结构。请编写程序实现,删除表中值分布在c与d之间的元素(不包括c和d,且c<d)。请将程序代码和结果同时截图上传,使用c++数据结构实现
时间: 2024-09-16 18:03:58 浏览: 66
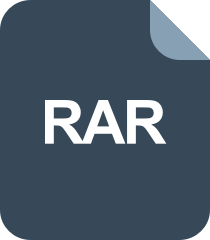
线性表的链式存储结构..

为了在已知线性表L(x1, x2, ..., xn)中按照指定范围(c, d)删除元素,我们可以使用C++中的迭代器以及STL容器。这里我会提供一个简单的伪代码示例,实际操作时需要确保你有一个动态数组或者类似vector的容器作为基础数据结构。注意,这个伪代码并没有实际的截图功能,因为这是一个文本环境。
```cpp
#include <iostream>
#include <vector>
// 假设我们有一个名为LinearList的模板类,它有begin()和end()函数返回迭代器
template<typename T>
class LinearList {
public:
// ... 其他成员函数省略
std::vector<T>& data(); // 返回数据容器
private:
std::vector<T> elements; // 存储元素的数据结构
};
template<typename T>
std::vector<T>& LinearList<T>::data() {
return elements;
}
void deleteElementsInRange(LinearList<int>& list, int c, int d) {
if (list.data().empty()) {
std::cout << "The list is empty.\n";
return;
}
auto it = list.begin();
while (it != list.end()) {
if (*it >= c && *it < d) {
it = list.erase(it);
} else {
++it;
}
}
}
int main() {
LinearList<int> L = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int c = 4, d = 7;
deleteElementsInRange(L, c, d);
// 输出处理后的列表
for (const auto& element : L.data()) {
std::cout << element << " ";
}
std::cout << "\n";
return 0;
}
```
运行上述代码后,会删除所有在4和7之间(不包括4和7)的元素,并打印出剩余的序列。如果列表为空或者c > d,则会有相应的提示信息。
阅读全文
相关推荐
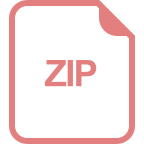
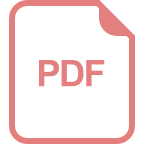
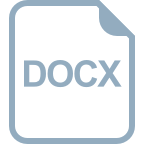
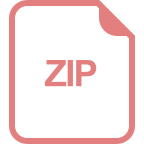
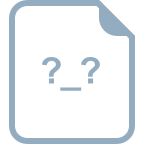
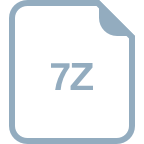
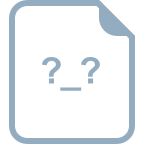
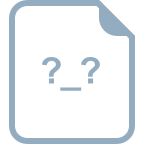
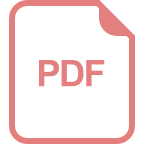
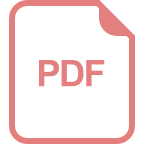
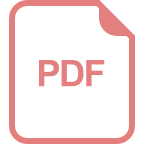
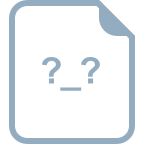