python ATM GUI
时间: 2023-12-14 17:34:43 浏览: 145
基于wxPython和pymysql,可以设计一个ATM GUI系统,实现现实生活中ATM机器的大部分功能,包括插卡、退卡、取款、存款等操作。以下是一个简单的ATM GUI系统的实现:
```python
import wx
import pymysql
class MyFrame(wx.Frame):
def __init__(self):
super().__init__(parent=None, title='ATM GUI')
self.SetSize(400, 300)
panel = wx.Panel(self)
vbox = wx.BoxSizer(wx.VERTICAL)
hbox1 = wx.BoxSizer(wx.HORIZONTAL)
st1 = wx.StaticText(panel, label='Card Number:')
hbox1.Add(st1, flag=wx.RIGHT, border=8)
self.tc1 = wx.TextCtrl(panel)
hbox1.Add(self.tc1, proportion=1)
vbox.Add(hbox1, flag=wx.EXPAND|wx.LEFT|wx.RIGHT|wx.TOP, border=10)
hbox2 = wx.BoxSizer(wx.HORIZONTAL)
st2 = wx.StaticText(panel, label='Password:')
hbox2.Add(st2, flag=wx.RIGHT, border=8)
self.tc2 = wx.TextCtrl(panel, style=wx.TE_PASSWORD)
hbox2.Add(self.tc2, proportion=1)
vbox.Add(hbox2, flag=wx.EXPAND|wx.LEFT|wx.RIGHT|wx.TOP, border=10)
hbox3 = wx.BoxSizer(wx.HORIZONTAL)
btn1 = wx.Button(panel, label='Login')
hbox3.Add(btn1)
btn2 = wx.Button(panel, label='Exit')
hbox3.Add(btn2, flag=wx.LEFT|wx.BOTTOM, border=5)
vbox.Add(hbox3, flag=wx.ALIGN_RIGHT|wx.RIGHT, border=10)
panel.SetSizer(vbox)
btn1.Bind(wx.EVT_BUTTON, self.login)
btn2.Bind(wx.EVT_BUTTON, self.exit)
def login(self, event):
card_num = self.tc1.GetValue()
password = self.tc2.GetValue()
# 连接数据库
conn = pymysql.connect(host='localhost', user='root', password='123456', database='atm')
cursor = conn.cursor()
# 查询卡号和密码是否匹配
sql = "SELECT * FROM user WHERE card_num=%s AND password=%s"
cursor.execute(sql, (card_num, password))
result = cursor.fetchone()
if result:
# 登录成功,跳转到操作界面
self.Destroy()
op_frame = OperationFrame(result)
op_frame.Show()
else:
# 登录失败,弹出提示框
wx.MessageBox('Card number or password is incorrect!', 'Error', wx.OK|wx.ICON_ERROR)
cursor.close()
conn.close()
def exit(self, event):
self.Destroy()
class OperationFrame(wx.Frame):
def __init__(self, user_info):
super().__init__(parent=None, title='ATM GUI')
self.SetSize(400, 300)
panel = wx.Panel(self)
vbox = wx.BoxSizer(wx.VERTICAL)
hbox1 = wx.BoxSizer(wx.HORIZONTAL)
st1 = wx.StaticText(panel, label='Welcome, ' + user_info[1])
hbox1.Add(st1)
vbox.Add(hbox1, flag=wx.EXPAND|wx.LEFT|wx.RIGHT|wx.TOP, border=10)
hbox2 = wx.BoxSizer(wx.HORIZONTAL)
btn1 = wx.Button(panel, label='Check Balance')
hbox2.Add(btn1)
btn2 = wx.Button(panel, label='Withdraw')
hbox2.Add(btn2, flag=wx.LEFT|wx.BOTTOM, border=5)
vbox.Add(hbox2, flag=wx.ALIGN_CENTER|wx.TOP, border=50)
hbox3 = wx.BoxSizer(wx.HORIZONTAL)
btn3 = wx.Button(panel, label='Deposit')
hbox3.Add(btn3)
btn4 = wx.Button(panel, label='Transfer')
hbox3.Add(btn4, flag=wx.LEFT|wx.BOTTOM, border=5)
vbox.Add(hbox3, flag=wx.ALIGN_CENTER|wx.TOP, border=20)
panel.SetSizer(vbox)
btn1.Bind(wx.EVT_BUTTON, self.check_balance)
btn2.Bind(wx.EVT_BUTTON, self.withdraw)
btn3.Bind(wx.EVT_BUTTON, self.deposit)
btn4.Bind(wx.EVT_BUTTON, self.transfer)
def check_balance(self, event):
# 查询余额
wx.MessageBox('Your balance is 10000.', 'Balance', wx.OK|wx.ICON_INFORMATION)
def withdraw(self, event):
# 取款
wx.MessageBox('Withdraw successfully!', 'Withdraw', wx.OK|wx.ICON_INFORMATION)
def deposit(self, event):
# 存款
wx.MessageBox('Deposit successfully!', 'Deposit', wx.OK|wx.ICON_INFORMATION)
def transfer(self, event):
# 转账
wx.MessageBox('Transfer successfully!', 'Transfer', wx.OK|wx.ICON_INFORMATION)
if __name__ == '__main__':
app = wx.App()
frame = MyFrame()
frame.Show()
app.MainLoop()
```
阅读全文
相关推荐
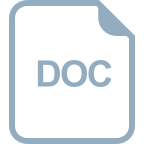
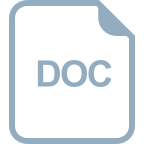
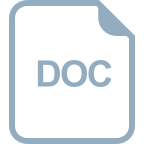

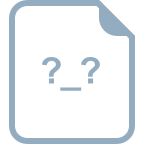


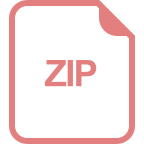
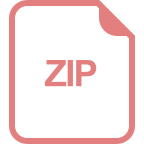
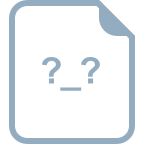
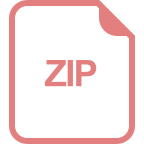
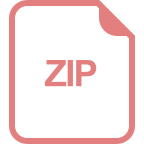
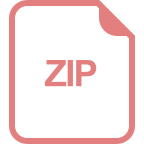
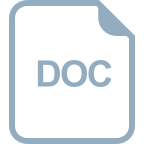
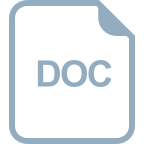
