MultipartFile 分片 Java实现
时间: 2023-10-17 07:34:00 浏览: 236
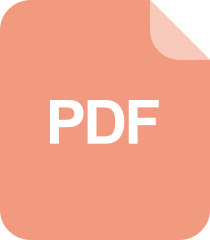
Java利用MultipartFile实现上传多份文件的代码
MultipartFile 分片上传是将一个大文件分成多个小的文件块进行上传,以减少网络传输中的丢包和传输错误的概率。以下是一个简单的 Java 实现示例:
1. 首先,确保你的项目中引入了相关的依赖库,例如 Spring 的 `spring-web` 和 Apache 的 `commons-io`。
2. 创建一个接收分片上传的控制器方法,示例代码如下:
```java
import org.apache.commons.io.FileUtils;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
@Controller
public class FileUploadController {
private static final String UPLOAD_DIR = "/path/to/upload/directory/";
@PostMapping("/upload")
public ResponseEntity<String> uploadFileChunk(
@RequestParam("file") MultipartFile file,
@RequestParam("chunkIndex") int chunkIndex,
@RequestParam("totalChunks") int totalChunks,
@RequestParam("filename") String filename) {
try {
// 创建分片上传目录
String uploadPath = UPLOAD_DIR + filename;
FileUtils.forceMkdir(new File(uploadPath));
// 保存分片文件
File chunkFile = new File(uploadPath, filename + "." + chunkIndex);
FileUtils.writeByteArrayToFile(chunkFile, file.getBytes());
// 判断是否已经上传完所有分片
if (chunkIndex == totalChunks - 1) {
// 合并分片文件
File mergedFile = new File(UPLOAD_DIR, filename);
for (int i = 0; i < totalChunks; i++) {
File partFile = new File(uploadPath, filename + "." + i);
FileUtils.writeByteArrayToFile(mergedFile, FileUtils.readFileToByteArray(partFile), true);
FileUtils.deleteQuietly(partFile);
}
FileUtils.deleteQuietly(new File(uploadPath));
// 返回上传成功的响应
return ResponseEntity.ok("File uploaded successfully");
} else {
// 返回当前分片上传成功的响应
return ResponseEntity.ok("Chunk uploaded successfully");
}
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("Error occurred during file upload");
}
}
}
```
3. 在上述代码中,`UPLOAD_DIR` 是用于保存上传文件的目录路径,你需要根据实际情况进行修改。
4. 在前端,你需要将大文件分成多个小块,并按照顺序逐个上传。每个分片上传时需要提供 `chunkIndex`(当前分片的索引)、`totalChunks`(总分片数)、`filename`(文件名)等参数,同时将文件块作为 `file` 参数进行上传。
这就是一个简单的 MultipartFile 分片上传的 Java 实现示例。你可以根据自己的需求进行适当的修改和扩展。
阅读全文
相关推荐
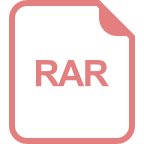
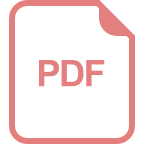





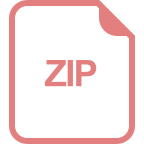
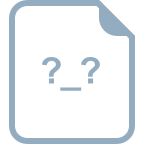
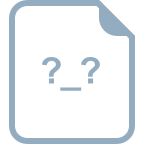
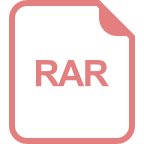
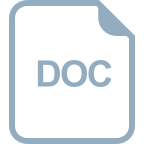





