bool serial_port::serial_port_write_read_at(const std::string &at_command, const std::string &resp_prefix, AT_RESPONSE *at_response) { char *read_line; bool ret_value = false; pthread_mutex_lock(&read_write_mutex); if (!serial_port_write_at_cmd(at_command.c_str())) { goto END; } do { read_line = serial_port_read_one_line(); if (read_line) { //LOG_F(INFO, "read one line from serial: %s", read_line); if (!strncmp(read_line, "AT", strlen("AT")) || !strncmp(read_line, "at", strlen("at"))) { //Do nothing } else if (!strncmp(read_line, "OK", strlen("OK"))) { //LOG_F(INFO, "find final response OK"); ret_value = true; if (at_response != nullptr) { at_response->state = RESP_OK; } break; } else if (!strncmp(read_line, "ERROR", strlen("ERROR")) || !strncmp(read_line, "+CME ERROR", strlen("+CME ERROR"))) { //LOG_F(INFO, "find final response ERROR"); ret_value = false; if (at_response != nullptr) { at_response->state = RESP_ERROR; } break; } else { if (at_response != nullptr) { //LOG_F(INFO, "put response string to AT_RESPONSE"); at_response->response_data[at_response->response_lines] = strdup(read_line); at_response->response_lines += 1; if (at_response->response_lines >= MAX_RESPONSE_LENGTH) at_response->response_lines = 0; } } } } while (true); END: pthread_mutex_unlock(&read_write_mutex); return ret_value; } 这段代码执行一遍没有跳出循环后,第二次开始执行是从哪里开始的,会再读一次serial_port_read_one_line吗
时间: 2024-04-18 14:30:51 浏览: 60
在第二次开始执行时,代码会再次执行serial_port_read_one_line函数。这是因为循环条件是`while (true)`,所以只要没有遇到`break`语句,循环会一直执行下去。每次循环开始时都会调用`serial_port_read_one_line`函数来读取一行数据,并根据读取的内容进行相应的处理。
相关问题
error: cannot convert ‘DceHelper::GlobalSeatFilter’ from type ‘bool (DceHelper::)(const string&, const string&, std::string&, int, int, bool) {aka bool (DceHelper::)(const std::basic_string<char>&, const std::basic_string<char>&, std::basic_string<char>&, int, int, bool)}’ to type ‘bool (*)(const string&, const string&, std::string&, int, int, bool) {aka bool (*)(const std::basic_string<char>&, const std::basic_string<char>&, std::basic_string<char>&, int, int, bool)}’
该错误提示表明不能将类型为“bool (DceHelper::)(const string&, const string&, std::string&, int, int, bool)”的成员函数指针转换为类型为“bool (*)(const string&, const string&, std::string&, int, int, bool)”的自由函数指针。
这是因为成员函数指针与自由函数指针是不同类型的。成员函数指针需要指定类的作用域,并且需要一个对象来调用该函数。而自由函数指针不需要指定类的作用域,也不需要对象来调用该函数。
如果您需要将成员函数指针转换为自由函数指针,则需要使用“std::bind”或“boost::bind”等函数绑定该成员函数的对象。例如,假设您有以下成员函数:
```
class MyClass {
public:
bool myFunction(const string& str);
};
```
您可以使用“std::bind”如下所示绑定该函数的对象,并将其转换为自由函数指针:
```
MyClass obj;
auto funcPtr = std::bind(&MyClass::myFunction, &obj, std::placeholders::_1);
bool (*freeFuncPtr)(const string&) = funcPtr;
```
在这个例子中,“std::bind”函数将“&MyClass::myFunction”和“&obj”作为参数来创建一个可调用对象,该对象可以像自由函数一样使用。然后,使用“auto”类型定义一个变量来存储可调用对象,并将其转换为自由函数指针。
注意,这里使用了“std::placeholders::_1”来表示该函数的第一个参数。如果该函数有多个参数,则需要使用“std::placeholders::_2”、“std::placeholders::_3”等来表示其他参数。
bool serial_port::serial_port_write_read_at(const std::string &at_command, const std::string &resp_prefix, AT_RESPONSE *at_response) { char *read_line; bool ret_value = false; pthread_mutex_lock(&read_write_mutex); if (!serial_port_write_at_cmd(at_command.c_str())) { goto END; } do { read_line = serial_port_read_one_line(); if (read_line) { //LOG_F(INFO, "read one line from serial: %s", read_line); if (!strncmp(read_line, "AT", strlen("AT")) || !strncmp(read_line, "at", strlen("at"))) { //Do nothing } else if (!strncmp(read_line, "OK", strlen("OK"))) { //LOG_F(INFO, "find final response OK"); ret_value = true; if (at_response != nullptr) { at_response->state = RESP_OK; } break; } else if (!strncmp(read_line, "ERROR", strlen("ERROR")) || !strncmp(read_line, "+CME ERROR", strlen("+CME ERROR"))) { //LOG_F(INFO, "find final response ERROR"); ret_value = false; if (at_response != nullptr) { at_response->state = RESP_ERROR; } break; } else { if (at_response != nullptr) { //LOG_F(INFO, "put response string to AT_RESPONSE"); at_response->response_data[at_response->response_lines] = strdup(read_line); at_response->response_lines += 1; if (at_response->response_lines >= MAX_RESPONSE_LENGTH) at_response->response_lines = 0; } } } } while (true); END: pthread_mutex_unlock(&read_write_mutex); return ret_value; } 没有break反复循环的情况下,开头都是从serial_port_read_one_line这里开始吗?也就是do后面开始执行
是的,如果没有`break`语句来跳出循环,那么每次循环开始时都会执行`serial_port_read_one_line`函数来读取一行数据,并根据读取的内容进行相应的处理。所以,在没有`break`的情况下,循环会一直执行,并且每次循环开始都会重新调用`serial_port_read_one_line`函数。在这段代码中,循环体的开头就是从`serial_port_read_one_line`这里开始执行。
阅读全文
相关推荐
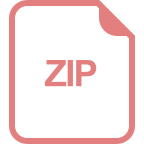
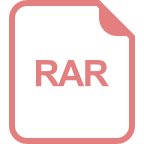


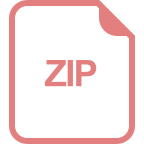
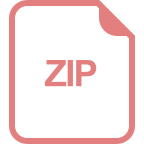
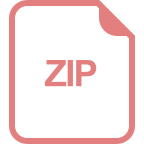
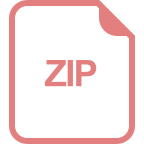
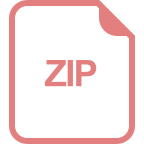
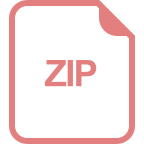
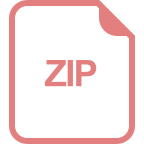
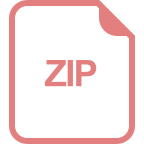
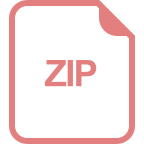
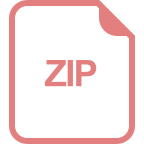
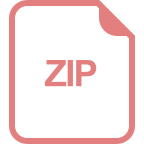
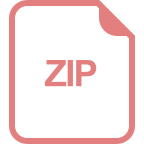